mirror of
https://github.com/git/git
synced 2024-10-28 19:25:47 +00:00
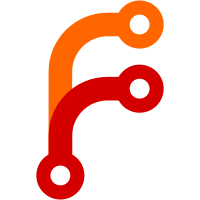
This is meant to make raw git not hugely less usable than something like raw CVS. I want to make a 1.0 release of the plumbing, and the actual commit part was just too intimidating.
48 lines
786 B
C
48 lines
786 B
C
#include <stdio.h>
|
|
#include <string.h>
|
|
#include <ctype.h>
|
|
|
|
/*
|
|
* Remove empty lines from the beginning and end.
|
|
*
|
|
* Turn multiple consecutive empty lines into just one
|
|
* empty line.
|
|
*/
|
|
static void cleanup(char *line)
|
|
{
|
|
int len = strlen(line);
|
|
|
|
if (len > 1 && line[len-1] == '\n') {
|
|
do {
|
|
unsigned char c = line[len-2];
|
|
if (!isspace(c))
|
|
break;
|
|
line[len-2] = '\n';
|
|
len--;
|
|
line[len] = 0;
|
|
} while (len > 1);
|
|
}
|
|
}
|
|
|
|
int main(int argc, char **argv)
|
|
{
|
|
int empties = -1;
|
|
char line[1024];
|
|
|
|
while (fgets(line, sizeof(line), stdin)) {
|
|
cleanup(line);
|
|
|
|
/* Not just an empty line? */
|
|
if (line[0] != '\n') {
|
|
if (empties > 0)
|
|
putchar('\n');
|
|
empties = 0;
|
|
fputs(line, stdout);
|
|
continue;
|
|
}
|
|
if (empties < 0)
|
|
continue;
|
|
empties++;
|
|
}
|
|
return 0;
|
|
}
|