mirror of
https://github.com/git/git
synced 2024-10-03 23:29:36 +00:00
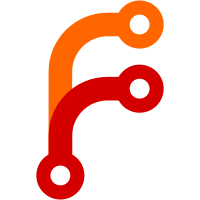
Add a helper function to make it clearer that retrieving 'update-clone' configuration from the .gitmodules file is a special case supported solely for backward compatibility purposes. This change removes one direct use of 'config_from_gitmodules' for options not strictly related to submodules: "submodule.fetchjobs" does not describe a property of a submodule, but a behavior of other commands when dealing with submodules, so it does not really belong to the .gitmodules file. This is in the effort to communicate better that .gitmodules is not to be used as a mechanism to store arbitrary configuration in the repository that any command can retrieve. Signed-off-by: Antonio Ospite <ao2@ao2.it> Acked-by: Brandon Williams <bmwill@google.com> Signed-off-by: Junio C Hamano <gitster@pobox.com>
73 lines
2.4 KiB
C
73 lines
2.4 KiB
C
#ifndef SUBMODULE_CONFIG_CACHE_H
|
|
#define SUBMODULE_CONFIG_CACHE_H
|
|
|
|
#include "cache.h"
|
|
#include "config.h"
|
|
#include "hashmap.h"
|
|
#include "submodule.h"
|
|
#include "strbuf.h"
|
|
|
|
/*
|
|
* Submodule entry containing the information about a certain submodule
|
|
* in a certain revision.
|
|
*/
|
|
struct submodule {
|
|
const char *path;
|
|
const char *name;
|
|
const char *url;
|
|
int fetch_recurse;
|
|
const char *ignore;
|
|
const char *branch;
|
|
struct submodule_update_strategy update_strategy;
|
|
/* the object id of the responsible .gitmodules file */
|
|
struct object_id gitmodules_oid;
|
|
int recommend_shallow;
|
|
};
|
|
|
|
#define SUBMODULE_INIT { NULL, NULL, NULL, RECURSE_SUBMODULES_NONE, \
|
|
NULL, NULL, SUBMODULE_UPDATE_STRATEGY_INIT, { { 0 } }, -1 };
|
|
|
|
struct submodule_cache;
|
|
struct repository;
|
|
|
|
extern void submodule_cache_free(struct submodule_cache *cache);
|
|
|
|
extern int parse_submodule_fetchjobs(const char *var, const char *value);
|
|
extern int parse_fetch_recurse_submodules_arg(const char *opt, const char *arg);
|
|
struct option;
|
|
extern int option_fetch_parse_recurse_submodules(const struct option *opt,
|
|
const char *arg, int unset);
|
|
extern int parse_update_recurse_submodules_arg(const char *opt, const char *arg);
|
|
extern int parse_push_recurse_submodules_arg(const char *opt, const char *arg);
|
|
extern void repo_read_gitmodules(struct repository *repo);
|
|
extern void gitmodules_config_oid(const struct object_id *commit_oid);
|
|
const struct submodule *submodule_from_name(struct repository *r,
|
|
const struct object_id *commit_or_tree,
|
|
const char *name);
|
|
const struct submodule *submodule_from_path(struct repository *r,
|
|
const struct object_id *commit_or_tree,
|
|
const char *path);
|
|
void submodule_free(struct repository *r);
|
|
|
|
/*
|
|
* Returns 0 if the name is syntactically acceptable as a submodule "name"
|
|
* (e.g., that may be found in the subsection of a .gitmodules file) and -1
|
|
* otherwise.
|
|
*/
|
|
int check_submodule_name(const char *name);
|
|
|
|
/*
|
|
* Note: This function exists solely to maintain backward compatibility with
|
|
* 'fetch' and 'update_clone' storing configuration in '.gitmodules' and should
|
|
* NOT be used anywhere else.
|
|
*
|
|
* Runs the provided config function on the '.gitmodules' file found in the
|
|
* working directory.
|
|
*/
|
|
extern void config_from_gitmodules(config_fn_t fn, void *data);
|
|
|
|
extern void fetch_config_from_gitmodules(int *max_children, int *recurse_submodules);
|
|
extern void update_clone_config_from_gitmodules(int *max_jobs);
|
|
|
|
#endif /* SUBMODULE_CONFIG_H */
|