mirror of
https://github.com/git/git
synced 2024-11-04 16:17:49 +00:00
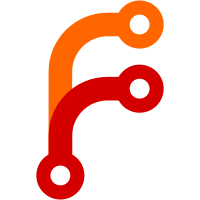
Memory allocation patterns in git-mv(1) are extremely hard to follow: We copy around string pointers into manually-managed arrays, some of which alias each other, but only sometimes, while we also drop some of those strings at other times without ever daring to free them. While this may be my own subjective feeling, it seems like others have given up as the code has multiple calls to `UNLEAK()`. These are not sufficient though, and git-mv(1) is still leaking all over the place even with them. Refactor the code to instead track strings in `struct strvec`. While this has the effect of effectively duplicating some of the strings without an actual need, it is way easier to reason about and fixes all of the aliasing of memory that has been going on. It allows us to get rid of the `UNLEAK()` calls and also fixes leaks that those calls did not paper over. Mark tests which are now leak-free accordingly. Signed-off-by: Patrick Steinhardt <ps@pks.im> Signed-off-by: Junio C Hamano <gitster@pobox.com>
107 lines
2.1 KiB
Bash
Executable file
107 lines
2.1 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
test_description='merging with large rename matrix'
|
|
GIT_TEST_DEFAULT_INITIAL_BRANCH_NAME=main
|
|
export GIT_TEST_DEFAULT_INITIAL_BRANCH_NAME
|
|
|
|
TEST_PASSES_SANITIZE_LEAK=true
|
|
. ./test-lib.sh
|
|
|
|
count() {
|
|
i=1
|
|
while test $i -le $1; do
|
|
echo $i
|
|
i=$(($i + 1))
|
|
done
|
|
}
|
|
|
|
test_expect_success 'setup (initial)' '
|
|
touch file &&
|
|
git add . &&
|
|
git commit -m initial &&
|
|
git tag initial
|
|
'
|
|
|
|
make_text() {
|
|
echo $1: $2
|
|
for i in $(count 20); do
|
|
echo $1: $i
|
|
done
|
|
echo $1: $3
|
|
}
|
|
|
|
test_rename() {
|
|
test_expect_success "rename ($1, $2)" '
|
|
n='$1' &&
|
|
expect='$2' &&
|
|
git checkout -f main &&
|
|
test_might_fail git branch -D test$n &&
|
|
git reset --hard initial &&
|
|
for i in $(count $n); do
|
|
make_text $i initial initial >$i || return 1
|
|
done &&
|
|
git add . &&
|
|
git commit -m add=$n &&
|
|
for i in $(count $n); do
|
|
make_text $i changed initial >$i || return 1
|
|
done &&
|
|
git commit -a -m change=$n &&
|
|
git checkout -b test$n HEAD^ &&
|
|
for i in $(count $n); do
|
|
git rm $i &&
|
|
make_text $i initial changed >$i.moved || return 1
|
|
done &&
|
|
git add . &&
|
|
git commit -m change+rename=$n &&
|
|
case "$expect" in
|
|
ok) git merge main ;;
|
|
*) test_must_fail git merge main ;;
|
|
esac
|
|
'
|
|
}
|
|
|
|
test_rename 5 ok
|
|
|
|
test_expect_success 'set diff.renamelimit to 4' '
|
|
git config diff.renamelimit 4
|
|
'
|
|
test_rename 4 ok
|
|
test_rename 5 fail
|
|
|
|
test_expect_success 'set merge.renamelimit to 5' '
|
|
git config merge.renamelimit 5
|
|
'
|
|
test_rename 5 ok
|
|
test_rename 6 fail
|
|
|
|
test_expect_success 'setup large simple rename' '
|
|
git config --unset merge.renamelimit &&
|
|
git config --unset diff.renamelimit &&
|
|
|
|
git reset --hard initial &&
|
|
for i in $(count 200); do
|
|
make_text foo bar baz >$i || return 1
|
|
done &&
|
|
git add . &&
|
|
git commit -m create-files &&
|
|
|
|
git branch simple-change &&
|
|
git checkout -b simple-rename &&
|
|
|
|
mkdir builtin &&
|
|
git mv [0-9]* builtin/ &&
|
|
git commit -m renamed &&
|
|
|
|
git checkout simple-change &&
|
|
>unrelated-change &&
|
|
git add unrelated-change &&
|
|
git commit -m unrelated-change
|
|
'
|
|
|
|
test_expect_success 'massive simple rename does not spam added files' '
|
|
sane_unset GIT_MERGE_VERBOSITY &&
|
|
git merge --no-stat simple-rename | grep -v Removing >output &&
|
|
test_line_count -lt 5 output
|
|
'
|
|
|
|
test_done
|