mirror of
https://github.com/git/git
synced 2024-11-04 16:17:49 +00:00
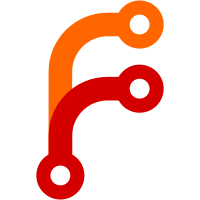
We leak the config values when `gpg_sign` or `strategy` options are being overridden via the command line. To fix this we need to free the old value, which requires us to figure out whether the value was changed via an option in the first place. The easy way to do this, which is to initialize local variables with `NULL`, doesn't work because we cannot tell the case where the user has passed e.g. `--no-gpg-sign`. Instead, we use a sentinel value for both values that we can compare against to check whether the user has passed the option. Signed-off-by: Patrick Steinhardt <ps@pks.im> Signed-off-by: Junio C Hamano <gitster@pobox.com>
87 lines
2.9 KiB
Bash
Executable file
87 lines
2.9 KiB
Bash
Executable file
#!/bin/sh
|
|
#
|
|
# Copyright (c) 2020 Doan Tran Cong Danh
|
|
#
|
|
|
|
test_description='test {cherry-pick,revert} --[no-]gpg-sign'
|
|
|
|
TEST_PASSES_SANITIZE_LEAK=true
|
|
. ./test-lib.sh
|
|
. "$TEST_DIRECTORY/lib-gpg.sh"
|
|
|
|
if ! test_have_prereq GPG
|
|
then
|
|
skip_all='skip all test {cherry-pick,revert} --[no-]gpg-sign, gpg not available'
|
|
test_done
|
|
fi
|
|
|
|
test_gpg_sign () {
|
|
local must_fail= will=will fake_editor=
|
|
if test "x$1" = "x!"
|
|
then
|
|
must_fail=test_must_fail
|
|
will="won't"
|
|
shift
|
|
fi
|
|
conf=$1
|
|
cmd=$2
|
|
cmit=$3
|
|
shift 3
|
|
test_expect_success "$cmd $* $cmit with commit.gpgsign=$conf $will sign commit" "
|
|
git reset --hard tip &&
|
|
git config commit.gpgsign $conf &&
|
|
git $cmd $* $cmit &&
|
|
git rev-list tip.. >rev-list &&
|
|
$must_fail git verify-commit \$(cat rev-list)
|
|
"
|
|
}
|
|
|
|
test_expect_success 'setup' '
|
|
test_commit one &&
|
|
git switch -c side &&
|
|
test_commit side1 &&
|
|
test_commit side2 &&
|
|
git switch - &&
|
|
test_commit two &&
|
|
test_commit three &&
|
|
test_commit tip
|
|
'
|
|
|
|
test_gpg_sign ! false cherry-pick side
|
|
test_gpg_sign ! false cherry-pick ..side
|
|
test_gpg_sign true cherry-pick side
|
|
test_gpg_sign true cherry-pick ..side
|
|
test_gpg_sign ! true cherry-pick side --no-gpg-sign
|
|
test_gpg_sign ! true cherry-pick ..side --no-gpg-sign
|
|
test_gpg_sign ! true cherry-pick side --gpg-sign --no-gpg-sign
|
|
test_gpg_sign ! true cherry-pick ..side --gpg-sign --no-gpg-sign
|
|
test_gpg_sign false cherry-pick side --no-gpg-sign --gpg-sign
|
|
test_gpg_sign false cherry-pick ..side --no-gpg-sign --gpg-sign
|
|
test_gpg_sign true cherry-pick side --edit
|
|
test_gpg_sign true cherry-pick ..side --edit
|
|
test_gpg_sign ! true cherry-pick side --edit --no-gpg-sign
|
|
test_gpg_sign ! true cherry-pick ..side --edit --no-gpg-sign
|
|
test_gpg_sign ! true cherry-pick side --edit --gpg-sign --no-gpg-sign
|
|
test_gpg_sign ! true cherry-pick ..side --edit --gpg-sign --no-gpg-sign
|
|
test_gpg_sign false cherry-pick side --edit --no-gpg-sign --gpg-sign
|
|
test_gpg_sign false cherry-pick ..side --edit --no-gpg-sign --gpg-sign
|
|
|
|
test_gpg_sign ! false revert HEAD --edit
|
|
test_gpg_sign ! false revert two.. --edit
|
|
test_gpg_sign true revert HEAD --edit
|
|
test_gpg_sign true revert two.. --edit
|
|
test_gpg_sign ! true revert HEAD --edit --no-gpg-sign
|
|
test_gpg_sign ! true revert two.. --edit --no-gpg-sign
|
|
test_gpg_sign ! true revert HEAD --edit --gpg-sign --no-gpg-sign
|
|
test_gpg_sign ! true revert two.. --edit --gpg-sign --no-gpg-sign
|
|
test_gpg_sign false revert HEAD --edit --no-gpg-sign --gpg-sign
|
|
test_gpg_sign false revert two.. --edit --no-gpg-sign --gpg-sign
|
|
test_gpg_sign true revert HEAD --no-edit
|
|
test_gpg_sign true revert two.. --no-edit
|
|
test_gpg_sign ! true revert HEAD --no-edit --no-gpg-sign
|
|
test_gpg_sign ! true revert two.. --no-edit --no-gpg-sign
|
|
test_gpg_sign ! true revert HEAD --no-edit --gpg-sign --no-gpg-sign
|
|
test_gpg_sign ! true revert two.. --no-edit --gpg-sign --no-gpg-sign
|
|
test_gpg_sign false revert HEAD --no-edit --no-gpg-sign --gpg-sign
|
|
|
|
test_done
|