mirror of
https://github.com/git/git
synced 2024-11-05 01:58:18 +00:00
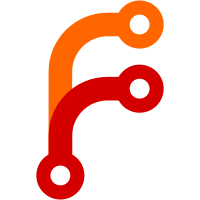
Our in-tree SHA-1 wrappers all define platform_SHA_CTX and related macros to point at the opaque "context" type, init, update, and similar functions for each specific implementation. In hash.h, we use these platform_ variables to set up the function pointers for, e.g., the_hash_algo->init_fn(), etc. But while these header files have a header-specific macro that prevents them declaring their structs / functions multiple times, they unconditionally define the platform variables, making it impossible to load multiple SHA-1 implementations at once. As a prerequisite for loading a separate SHA-1 implementation for non-cryptographic uses, only define the platform_ variables if they have not already been defined. Signed-off-by: Taylor Blau <me@ttaylorr.com> Signed-off-by: Junio C Hamano <gitster@pobox.com>
51 lines
1.2 KiB
C
51 lines
1.2 KiB
C
/* wrappers for the EVP API of OpenSSL 3+ */
|
|
#ifndef SHA1_OPENSSL_H
|
|
#define SHA1_OPENSSL_H
|
|
#include <openssl/evp.h>
|
|
|
|
struct openssl_SHA1_CTX {
|
|
EVP_MD_CTX *ectx;
|
|
};
|
|
|
|
typedef struct openssl_SHA1_CTX openssl_SHA1_CTX;
|
|
|
|
static inline void openssl_SHA1_Init(struct openssl_SHA1_CTX *ctx)
|
|
{
|
|
const EVP_MD *type = EVP_sha1();
|
|
|
|
ctx->ectx = EVP_MD_CTX_new();
|
|
if (!ctx->ectx)
|
|
die("EVP_MD_CTX_new: out of memory");
|
|
|
|
EVP_DigestInit_ex(ctx->ectx, type, NULL);
|
|
}
|
|
|
|
static inline void openssl_SHA1_Update(struct openssl_SHA1_CTX *ctx,
|
|
const void *data,
|
|
size_t len)
|
|
{
|
|
EVP_DigestUpdate(ctx->ectx, data, len);
|
|
}
|
|
|
|
static inline void openssl_SHA1_Final(unsigned char *digest,
|
|
struct openssl_SHA1_CTX *ctx)
|
|
{
|
|
EVP_DigestFinal_ex(ctx->ectx, digest, NULL);
|
|
EVP_MD_CTX_free(ctx->ectx);
|
|
}
|
|
|
|
static inline void openssl_SHA1_Clone(struct openssl_SHA1_CTX *dst,
|
|
const struct openssl_SHA1_CTX *src)
|
|
{
|
|
EVP_MD_CTX_copy_ex(dst->ectx, src->ectx);
|
|
}
|
|
|
|
#ifndef platform_SHA_CTX
|
|
#define platform_SHA_CTX openssl_SHA1_CTX
|
|
#define platform_SHA1_Init openssl_SHA1_Init
|
|
#define platform_SHA1_Clone openssl_SHA1_Clone
|
|
#define platform_SHA1_Update openssl_SHA1_Update
|
|
#define platform_SHA1_Final openssl_SHA1_Final
|
|
#endif
|
|
|
|
#endif /* SHA1_OPENSSL_H */
|