mirror of
https://github.com/git/git
synced 2024-11-04 16:17:49 +00:00
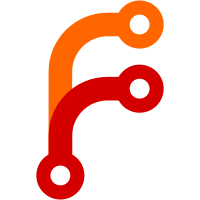
The reftable library does not use the same memory allocation functions as the rest of the Git codebase. Instead, as the reftable library is supposed to be usable as a standalone library without Git, it provides a set of pluggable memory allocators. Compared to `xmalloc()` and friends these allocators are _not_ expected to die when an allocation fails. This design choice is concious, as a library should leave it to its caller to handle any kind of error. While it is very likely that the caller cannot really do much in the case of an out-of-memory situation anyway, we are not the ones to make that decision. Curiously though, we never handle allocation errors even though memory allocation functions are allowed to fail. And as we do not plug in Git's memory allocator via `reftable_set_alloc()` either the consequence is that we'd instead segfault as soon as we run out of memory. While the easy fix would be to wire up `xmalloc()` and friends, it would only fix the usage of the reftable library in Git itself. Other users like libgit2, which is about to revive its efforts to land a backend for reftables, wouldn't be able to benefit from this solution. Instead, we are about to do it the hard way: adapt all allocation sites to perform error checking. Introduce a new error code for out-of-memory errors that we will wire up in subsequent steps. This commit also serves as the motivator for all the remaining steps in this series such that we do not have to repeat the same arguments in every single subsequent commit. Signed-off-by: Patrick Steinhardt <ps@pks.im> Signed-off-by: Junio C Hamano <gitster@pobox.com>
69 lines
1.8 KiB
C
69 lines
1.8 KiB
C
/*
|
|
Copyright 2020 Google LLC
|
|
|
|
Use of this source code is governed by a BSD-style
|
|
license that can be found in the LICENSE file or at
|
|
https://developers.google.com/open-source/licenses/bsd
|
|
*/
|
|
|
|
#ifndef REFTABLE_ERROR_H
|
|
#define REFTABLE_ERROR_H
|
|
|
|
/*
|
|
* Errors in reftable calls are signaled with negative integer return values. 0
|
|
* means success.
|
|
*/
|
|
enum reftable_error {
|
|
/* Unexpected file system behavior */
|
|
REFTABLE_IO_ERROR = -2,
|
|
|
|
/* Format inconsistency on reading data */
|
|
REFTABLE_FORMAT_ERROR = -3,
|
|
|
|
/* File does not exist. Returned from block_source_from_file(), because
|
|
* it needs special handling in stack.
|
|
*/
|
|
REFTABLE_NOT_EXIST_ERROR = -4,
|
|
|
|
/* Trying to access locked data. */
|
|
REFTABLE_LOCK_ERROR = -5,
|
|
|
|
/* Misuse of the API:
|
|
* - on writing a record with NULL refname.
|
|
* - on writing a reftable_ref_record outside the table limits
|
|
* - on writing a ref or log record before the stack's
|
|
* next_update_inde*x
|
|
* - on writing a log record with multiline message with
|
|
* exact_log_message unset
|
|
* - on reading a reftable_ref_record from log iterator, or vice versa.
|
|
*
|
|
* When a call misuses the API, the internal state of the library is
|
|
* kept unchanged.
|
|
*/
|
|
REFTABLE_API_ERROR = -6,
|
|
|
|
/* Decompression error */
|
|
REFTABLE_ZLIB_ERROR = -7,
|
|
|
|
/* Wrote a table without blocks. */
|
|
REFTABLE_EMPTY_TABLE_ERROR = -8,
|
|
|
|
/* Invalid ref name. */
|
|
REFTABLE_REFNAME_ERROR = -10,
|
|
|
|
/* Entry does not fit. This can happen when writing outsize reflog
|
|
messages. */
|
|
REFTABLE_ENTRY_TOO_BIG_ERROR = -11,
|
|
|
|
/* Trying to write out-of-date data. */
|
|
REFTABLE_OUTDATED_ERROR = -12,
|
|
|
|
/* An allocation has failed due to an out-of-memory situation. */
|
|
REFTABLE_OUT_OF_MEMORY_ERROR = -13,
|
|
};
|
|
|
|
/* convert the numeric error code to a string. The string should not be
|
|
* deallocated. */
|
|
const char *reftable_error_str(int err);
|
|
|
|
#endif
|