mirror of
https://github.com/flutter/flutter
synced 2024-11-05 18:37:51 +00:00
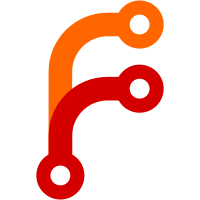
Context: https://github.com/flutter/flutter/issues/131862 This PR injects a "realm" component to the storage base URL when the contents of the file `bin/internal/engine.realm` is non-empty. As documented in the PR, when the realm is `flutter_archives_v2`, and `bin/internal/engine.version` contains the commit hash for a commit in a `flutter/engine` PR, then the artifacts pulled by the tool will be the artifacts built by the presubmit checks for the PR. This works for everything but the following two cases: 1. Fuchsia artifacts are not uploaded to CIPD by the Fuchsia presubmit builds. 2. Web artifacts are not uploaded to gstatic by the web engine presubmit builds. For (1), the flutter/flutter presubmit `fuchsia_precache` is driven by a shell script outside of the repo. It will fail when the `engine.version` and `engine.realm` don't point to a post-submit engine commit. For (2), the flutter/flutter web presubmit tests that refer to artifacts in gstatic hang when the artifacts aren't found, so this PR skips them.
96 lines
3.3 KiB
Dart
96 lines
3.3 KiB
Dart
// Copyright 2014 The Flutter Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style license that can be
|
|
// found in the LICENSE file.
|
|
|
|
import 'dart:convert';
|
|
import 'dart:io';
|
|
|
|
import 'package:flutter_devicelab/framework/apk_utils.dart';
|
|
import 'package:flutter_devicelab/framework/framework.dart';
|
|
import 'package:flutter_devicelab/framework/task_result.dart';
|
|
import 'package:flutter_devicelab/framework/utils.dart';
|
|
import 'package:path/path.dart' as path;
|
|
|
|
final String gradlew = Platform.isWindows ? 'gradlew.bat' : 'gradlew';
|
|
final String gradlewExecutable = Platform.isWindows ? '.\\$gradlew' : './$gradlew';
|
|
|
|
/// Tests that we respect storage proxy URLs in gradle dependencies.
|
|
Future<void> main() async {
|
|
await task(() async {
|
|
section('Find Java');
|
|
|
|
final String? javaHome = await findJavaHome();
|
|
if (javaHome == null) {
|
|
return TaskResult.failure('Could not find Java');
|
|
}
|
|
print('\nUsing JAVA_HOME=$javaHome');
|
|
|
|
section('Create project');
|
|
await runProjectTest((FlutterProject flutterProject) async {
|
|
await inDirectory(path.join(flutterProject.rootPath, 'android'), () async {
|
|
section('Insert gradle testing script');
|
|
final File build = File(path.join(
|
|
flutterProject.rootPath, 'android', 'app', 'build.gradle',
|
|
));
|
|
build.writeAsStringSync(
|
|
'''
|
|
task printEngineMavenUrl() {
|
|
doLast {
|
|
println project.repositories.find { it.name == 'maven' }.url
|
|
}
|
|
}
|
|
''',
|
|
mode: FileMode.append,
|
|
flush: true,
|
|
);
|
|
|
|
section('Checking default maven URL');
|
|
|
|
String gradleOutput = await eval(
|
|
gradlewExecutable,
|
|
<String>['printEngineMavenUrl', '-q'],
|
|
);
|
|
const LineSplitter splitter = LineSplitter();
|
|
List<String> outputLines = splitter.convert(gradleOutput);
|
|
String mavenUrl = outputLines.last;
|
|
print('Returned maven url: $mavenUrl');
|
|
|
|
String realm = File(
|
|
path.join(flutterDirectory.path, 'bin', 'internal', 'engine.realm'),
|
|
).readAsStringSync().trim();
|
|
if (realm.isNotEmpty) {
|
|
realm = '$realm/';
|
|
}
|
|
|
|
if (mavenUrl != 'https://storage.googleapis.com/${realm}download.flutter.io') {
|
|
throw TaskResult.failure(
|
|
'Expected Android engine maven dependency URL to '
|
|
'resolve to https://storage.googleapis.com/${realm}download.flutter.io. Got '
|
|
'$mavenUrl instead',
|
|
);
|
|
}
|
|
|
|
section('Checking overridden maven URL');
|
|
gradleOutput = await eval(
|
|
gradlewExecutable,
|
|
<String>['printEngineMavenUrl','-q'],
|
|
environment: <String, String>{
|
|
'FLUTTER_STORAGE_BASE_URL': 'https://my.special.proxy',
|
|
},
|
|
);
|
|
outputLines = splitter.convert(gradleOutput);
|
|
mavenUrl = outputLines.last;
|
|
|
|
if (mavenUrl != 'https://my.special.proxy/${realm}download.flutter.io') {
|
|
throw TaskResult.failure(
|
|
'Expected overridden Android engine maven '
|
|
'dependency URL to resolve to proxy location '
|
|
'https://my.special.proxy/${realm}download.flutter.io. Got '
|
|
'$mavenUrl instead',
|
|
);
|
|
}
|
|
});
|
|
});
|
|
return TaskResult.success(null);
|
|
});
|
|
}
|