mirror of
https://github.com/flutter/flutter
synced 2024-10-13 19:52:53 +00:00
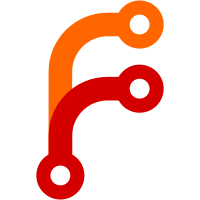
This converts all remaining "## Sample code" segments into snippets, and fixes the snippet generator to handle multiple snippets in the same dartdoc block properly. I also generated, compiled, and ran each of the existing application samples, and fixed them up to be more useful and/or just run without errors. This PR fixes these problems with examples: 1. Switching tabs in a snippet now works if there is more than one snippet in a single dartdoc block. 2. Generation of snippet code now works if there is more than one snippet. 3. Contrast of text and links in the code sample block has been improved to recommended levels. 4. Added five new snippet templates, including a "freeform" template to make it possible to show examples that need to change the app instantiation. 5. Fixed several examples to run properly, a couple by adding the "Scaffold" widget to the template, a couple by just fixing their code. 6. Fixed visual look of some of the samples when they run by placing many samples inside of a Scaffold. 7. In order to make it easier to run locally, changed the sample analyzer to remove the contents of the supplied temp directory before running, since having files that hang around is problematic (only a problem when running locally with the `--temp` argument). 8. Added a `SampleCheckerException` class, and handle sample checking exceptions more gracefully. 9. Deprecated the old "## Sample code" designation, and added enforcement for the deprecation. 10. Removed unnecessary `new` from templates (although they never appeared in the samples thanks to dartfmt, but still). Fixes #26398 Fixes #27411
90 lines
2.5 KiB
JavaScript
90 lines
2.5 KiB
JavaScript
/**
|
|
* Scripting for handling custom code snippets
|
|
*/
|
|
|
|
/**
|
|
* Shows the requested snippet, and stores the current state in visibleSnippet.
|
|
*/
|
|
function showSnippet(name, visibleSnippet) {
|
|
if (visibleSnippet == name) return;
|
|
if (visibleSnippet != null) {
|
|
var shown = document.getElementById(visibleSnippet);
|
|
var attribute = document.createAttribute('hidden');
|
|
if (shown != null) {
|
|
shown.setAttributeNode(attribute);
|
|
}
|
|
var button = document.getElementById(visibleSnippet + 'Button');
|
|
if (button != null) {
|
|
button.removeAttribute('selected');
|
|
}
|
|
}
|
|
if (name == null || name == '') {
|
|
visibleSnippet = null;
|
|
return;
|
|
}
|
|
var newlyVisible = document.getElementById(name);
|
|
if (newlyVisible != null) {
|
|
visibleSnippet = name;
|
|
newlyVisible.removeAttribute('hidden');
|
|
} else {
|
|
visibleSnippet = null;
|
|
}
|
|
var button = document.getElementById(name + 'Button');
|
|
var selectedAttribute = document.createAttribute('selected');
|
|
if (button != null) {
|
|
button.setAttributeNode(selectedAttribute);
|
|
}
|
|
return visibleSnippet;
|
|
}
|
|
|
|
// Finds a sibling to given element with the given id.
|
|
function findSiblingWithId(element, id) {
|
|
var siblings = element.parentNode.children;
|
|
var siblingWithId = null;
|
|
for (var i = siblings.length; i--;) {
|
|
if (siblings[i] == element) continue;
|
|
if (siblings[i].id == id) {
|
|
siblingWithId = siblings[i];
|
|
break;
|
|
}
|
|
}
|
|
return siblingWithId;
|
|
};
|
|
|
|
// Returns true if the browser supports the "copy" command.
|
|
function supportsCopying() {
|
|
return !!document.queryCommandSupported &&
|
|
!!document.queryCommandSupported('copy');
|
|
}
|
|
|
|
// Copies the text inside the currently visible snippet to the clipboard, or the
|
|
// given element, if any.
|
|
function copyTextToClipboard(element) {
|
|
if (typeof element === 'string') {
|
|
var elementSelector = '#' + element + ' .language-dart';
|
|
element = document.querySelector(elementSelector);
|
|
if (element == null) {
|
|
console.log(
|
|
'copyTextToClipboard: Unable to find element for "' +
|
|
elementSelector + '"');
|
|
return;
|
|
}
|
|
}
|
|
if (!supportsCopying()) {
|
|
alert('Unable to copy to clipboard (not supported by browser)');
|
|
return;
|
|
}
|
|
|
|
if (element.hasAttribute('contenteditable')) {
|
|
element.focus();
|
|
}
|
|
|
|
var selection = window.getSelection();
|
|
var range = document.createRange();
|
|
|
|
range.selectNodeContents(element);
|
|
selection.removeAllRanges();
|
|
selection.addRange(range);
|
|
document.execCommand('copy');
|
|
}
|