mirror of
https://github.com/flutter/flutter
synced 2024-10-13 19:52:53 +00:00
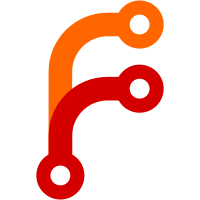
* Update project.pbxproj files to say Flutter rather than Chromium Also, the templates now have an empty organization so that we don't cause people to give their apps a Flutter copyright. * Update the copyright notice checker to require a standard notice on all files * Update copyrights on Dart files. (This was a mechanical commit.) * Fix weird license headers on Dart files that deviate from our conventions; relicense Shrine. Some were already marked "The Flutter Authors", not clear why. Their dates have been normalized. Some were missing the blank line after the license. Some were randomly different in trivial ways for no apparent reason (e.g. missing the trailing period). * Clean up the copyrights in non-Dart files. (Manual edits.) Also, make sure templates don't have copyrights. * Fix some more ORGANIZATIONNAMEs
174 lines
7.5 KiB
Dart
174 lines
7.5 KiB
Dart
// Copyright 2014 The Flutter Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style license that can be
|
|
// found in the LICENSE file.
|
|
|
|
import 'package:flutter_tools/src/build_info.dart';
|
|
import 'package:flutter_tools/src/base/common.dart';
|
|
import 'package:flutter_tools/src/base/io.dart';
|
|
import 'package:flutter_tools/src/base/platform.dart';
|
|
import 'package:flutter_tools/src/test/flutter_platform.dart';
|
|
import 'package:meta/meta.dart';
|
|
|
|
import 'package:mockito/mockito.dart';
|
|
import 'package:process/process.dart';
|
|
import 'package:test_core/backend.dart'; // ignore: deprecated_member_use
|
|
|
|
import '../src/common.dart';
|
|
import '../src/context.dart';
|
|
|
|
void main() {
|
|
group('FlutterPlatform', () {
|
|
testUsingContext('ensureConfiguration throws an error if an explicitObservatoryPort is specified and more than one test file', () async {
|
|
final FlutterPlatform flutterPlatform = FlutterPlatform(buildMode: BuildMode.debug, shellPath: '/', explicitObservatoryPort: 1234);
|
|
flutterPlatform.loadChannel('test1.dart', MockSuitePlatform());
|
|
expect(() => flutterPlatform.loadChannel('test2.dart', MockSuitePlatform()), throwsA(isA<ToolExit>()));
|
|
});
|
|
|
|
testUsingContext('ensureConfiguration throws an error if a precompiled entrypoint is specified and more that one test file', () {
|
|
final FlutterPlatform flutterPlatform = FlutterPlatform(buildMode: BuildMode.debug, shellPath: '/', precompiledDillPath: 'example.dill');
|
|
flutterPlatform.loadChannel('test1.dart', MockSuitePlatform());
|
|
expect(() => flutterPlatform.loadChannel('test2.dart', MockSuitePlatform()), throwsA(isA<ToolExit>()));
|
|
});
|
|
|
|
group('The FLUTTER_TEST environment variable is passed to the test process', () {
|
|
MockPlatform mockPlatform;
|
|
MockProcessManager mockProcessManager;
|
|
FlutterPlatform flutterPlatform;
|
|
final Map<Type, Generator> contextOverrides = <Type, Generator>{
|
|
Platform: () => mockPlatform,
|
|
ProcessManager: () => mockProcessManager,
|
|
};
|
|
|
|
setUp(() {
|
|
mockPlatform = MockPlatform();
|
|
mockProcessManager = MockProcessManager();
|
|
flutterPlatform = TestFlutterPlatform();
|
|
});
|
|
|
|
Future<Map<String, String>> captureEnvironment() async {
|
|
flutterPlatform.loadChannel('test1.dart', MockSuitePlatform());
|
|
await untilCalled(mockProcessManager.start(any, environment: anyNamed('environment')));
|
|
final VerificationResult toVerify = verify(mockProcessManager.start(any, environment: captureAnyNamed('environment')));
|
|
expect(toVerify.captured, hasLength(1));
|
|
expect(toVerify.captured.first, isInstanceOf<Map<String, String>>());
|
|
return toVerify.captured.first as Map<String, String>;
|
|
}
|
|
|
|
testUsingContext('as true when not originally set', () async {
|
|
when(mockPlatform.environment).thenReturn(<String, String>{});
|
|
final Map<String, String> capturedEnvironment = await captureEnvironment();
|
|
expect(capturedEnvironment['FLUTTER_TEST'], 'true');
|
|
}, overrides: contextOverrides);
|
|
|
|
testUsingContext('as true when set to true', () async {
|
|
when(mockPlatform.environment).thenReturn(<String, String>{'FLUTTER_TEST': 'true'});
|
|
final Map<String, String> capturedEnvironment = await captureEnvironment();
|
|
expect(capturedEnvironment['FLUTTER_TEST'], 'true');
|
|
}, overrides: contextOverrides);
|
|
|
|
testUsingContext('as false when set to false', () async {
|
|
when(mockPlatform.environment).thenReturn(<String, String>{'FLUTTER_TEST': 'false'});
|
|
final Map<String, String> capturedEnvironment = await captureEnvironment();
|
|
expect(capturedEnvironment['FLUTTER_TEST'], 'false');
|
|
}, overrides: contextOverrides);
|
|
|
|
testUsingContext('unchanged when set', () async {
|
|
when(mockPlatform.environment).thenReturn(<String, String>{'FLUTTER_TEST': 'neither true nor false'});
|
|
final Map<String, String> capturedEnvironment = await captureEnvironment();
|
|
expect(capturedEnvironment['FLUTTER_TEST'], 'neither true nor false');
|
|
}, overrides: contextOverrides);
|
|
|
|
testUsingContext('as null when set to null', () async {
|
|
when(mockPlatform.environment).thenReturn(<String, String>{'FLUTTER_TEST': null});
|
|
final Map<String, String> capturedEnvironment = await captureEnvironment();
|
|
expect(capturedEnvironment['FLUTTER_TEST'], null);
|
|
}, overrides: contextOverrides);
|
|
});
|
|
|
|
testUsingContext('installHook creates a FlutterPlatform', () {
|
|
expect(() => installHook(
|
|
buildMode: BuildMode.debug,
|
|
shellPath: 'abc',
|
|
enableObservatory: false,
|
|
startPaused: true,
|
|
), throwsA(isA<AssertionError>()));
|
|
|
|
expect(() => installHook(
|
|
buildMode: BuildMode.debug,
|
|
shellPath: 'abc',
|
|
enableObservatory: false,
|
|
startPaused: false,
|
|
observatoryPort: 123,
|
|
), throwsA(isA<AssertionError>()));
|
|
|
|
FlutterPlatform capturedPlatform;
|
|
final Map<String, String> expectedPrecompiledDillFiles = <String, String>{'Key': 'Value'};
|
|
final FlutterPlatform flutterPlatform = installHook(
|
|
shellPath: 'abc',
|
|
enableObservatory: true,
|
|
machine: true,
|
|
startPaused: true,
|
|
disableServiceAuthCodes: true,
|
|
port: 100,
|
|
precompiledDillPath: 'def',
|
|
precompiledDillFiles: expectedPrecompiledDillFiles,
|
|
buildMode: BuildMode.debug,
|
|
trackWidgetCreation: true,
|
|
updateGoldens: true,
|
|
buildTestAssets: true,
|
|
observatoryPort: 200,
|
|
serverType: InternetAddressType.IPv6,
|
|
icudtlPath: 'ghi',
|
|
platformPluginRegistration: (FlutterPlatform platform) {
|
|
capturedPlatform = platform;
|
|
});
|
|
|
|
expect(identical(capturedPlatform, flutterPlatform), equals(true));
|
|
expect(flutterPlatform.shellPath, equals('abc'));
|
|
expect(flutterPlatform.enableObservatory, equals(true));
|
|
expect(flutterPlatform.machine, equals(true));
|
|
expect(flutterPlatform.startPaused, equals(true));
|
|
expect(flutterPlatform.disableServiceAuthCodes, equals(true));
|
|
expect(flutterPlatform.port, equals(100));
|
|
expect(flutterPlatform.host, InternetAddress.loopbackIPv6);
|
|
expect(flutterPlatform.explicitObservatoryPort, equals(200));
|
|
expect(flutterPlatform.precompiledDillPath, equals('def'));
|
|
expect(flutterPlatform.precompiledDillFiles, expectedPrecompiledDillFiles);
|
|
expect(flutterPlatform.buildMode, equals(BuildMode.debug));
|
|
expect(flutterPlatform.trackWidgetCreation, equals(true));
|
|
expect(flutterPlatform.updateGoldens, equals(true));
|
|
expect(flutterPlatform.buildTestAssets, equals(true));
|
|
expect(flutterPlatform.icudtlPath, equals('ghi'));
|
|
});
|
|
});
|
|
}
|
|
|
|
class MockSuitePlatform extends Mock implements SuitePlatform {}
|
|
|
|
class MockProcessManager extends Mock implements ProcessManager {}
|
|
|
|
class MockPlatform extends Mock implements Platform {}
|
|
|
|
class MockHttpServer extends Mock implements HttpServer {}
|
|
|
|
// A FlutterPlatform with enough fields set to load and start a test.
|
|
//
|
|
// Uses a mock HttpServer. We don't want to bind random ports in our CI hosts.
|
|
class TestFlutterPlatform extends FlutterPlatform {
|
|
TestFlutterPlatform() : super(
|
|
buildMode: BuildMode.debug,
|
|
shellPath: '/',
|
|
precompiledDillPath: 'example.dill',
|
|
host: InternetAddress.loopbackIPv6,
|
|
port: 0,
|
|
updateGoldens: false,
|
|
startPaused: false,
|
|
enableObservatory: false,
|
|
buildTestAssets: false,
|
|
);
|
|
|
|
@override
|
|
@protected
|
|
Future<HttpServer> bind(InternetAddress host, int port) async => MockHttpServer();
|
|
}
|