mirror of
https://github.com/flutter/flutter
synced 2024-10-14 04:02:56 +00:00
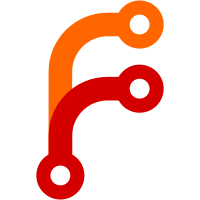
It was resulting in weird situations where the tool would dump an error message and stack but not quit, or would fail hard but then just hang. Instead, specifically catch errors you expect. As an example of this, there's one error we expect from the DartDependencySetBuilder, so we catch that one, turn it into a dedicated exception class, then in the caller catch that specific exception.
78 lines
3 KiB
Dart
78 lines
3 KiB
Dart
// Copyright 2016 The Chromium Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style license that can be
|
|
// found in the LICENSE file.
|
|
|
|
import 'package:flutter_tools/src/dart/dependencies.dart';
|
|
import 'package:flutter_tools/src/base/file_system.dart';
|
|
import 'package:test/test.dart';
|
|
|
|
import 'src/common.dart';
|
|
import 'src/context.dart';
|
|
|
|
void main() {
|
|
group('DartDependencySetBuilder', () {
|
|
final String dataPath = fs.path.join(
|
|
getFlutterRoot(),
|
|
'packages',
|
|
'flutter_tools',
|
|
'test',
|
|
'data',
|
|
'dart_dependencies_test',
|
|
);
|
|
|
|
testUsingContext('good', () {
|
|
final String testPath = fs.path.join(dataPath, 'good');
|
|
final String mainPath = fs.path.join(testPath, 'main.dart');
|
|
final String packagesPath = fs.path.join(testPath, '.packages');
|
|
final DartDependencySetBuilder builder =
|
|
new DartDependencySetBuilder(mainPath, packagesPath);
|
|
final Set<String> dependencies = builder.build();
|
|
expect(dependencies.contains(fs.path.canonicalize(mainPath)), isTrue);
|
|
expect(dependencies.contains(fs.path.canonicalize(fs.path.join(testPath, 'foo.dart'))), isTrue);
|
|
});
|
|
|
|
testUsingContext('syntax_error', () {
|
|
final String testPath = fs.path.join(dataPath, 'syntax_error');
|
|
final String mainPath = fs.path.join(testPath, 'main.dart');
|
|
final String packagesPath = fs.path.join(testPath, '.packages');
|
|
final DartDependencySetBuilder builder =
|
|
new DartDependencySetBuilder(mainPath, packagesPath);
|
|
try {
|
|
builder.build();
|
|
fail('expect an exception to be thrown.');
|
|
} on DartDependencyException catch (error) {
|
|
expect(error.toString(), contains('foo.dart: Expected a string literal'));
|
|
}
|
|
});
|
|
|
|
testUsingContext('bad_path', () {
|
|
final String testPath = fs.path.join(dataPath, 'bad_path');
|
|
final String mainPath = fs.path.join(testPath, 'main.dart');
|
|
final String packagesPath = fs.path.join(testPath, '.packages');
|
|
final DartDependencySetBuilder builder =
|
|
new DartDependencySetBuilder(mainPath, packagesPath);
|
|
try {
|
|
builder.build();
|
|
fail('expect an exception to be thrown.');
|
|
} on DartDependencyException catch (error) {
|
|
expect(error.toString(), contains('amaze${fs.path.separator}and${fs.path.separator}astonish.dart'));
|
|
}
|
|
});
|
|
|
|
testUsingContext('bad_package', () {
|
|
final String testPath = fs.path.join(dataPath, 'bad_package');
|
|
final String mainPath = fs.path.join(testPath, 'main.dart');
|
|
final String packagesPath = fs.path.join(testPath, '.packages');
|
|
final DartDependencySetBuilder builder =
|
|
new DartDependencySetBuilder(mainPath, packagesPath);
|
|
try {
|
|
builder.build();
|
|
fail('expect an exception to be thrown.');
|
|
} on DartDependencyException catch (error) {
|
|
expect(error.toString(), contains('rochambeau'));
|
|
expect(error.toString(), contains('pubspec.yaml'));
|
|
}
|
|
});
|
|
});
|
|
}
|