mirror of
https://github.com/flutter/flutter
synced 2024-08-27 12:00:58 +00:00
Modified TextField docs - Replaced 'labelText' to 'hintText' in code snippet (#94128)
Modified TextField docs - Replaced 'labelText' to 'hintText' in code snippet
This commit is contained in:
parent
c71f1dd76b
commit
f44064991d
42
examples/api/lib/material/text_field/text_field.0.dart
Normal file
42
examples/api/lib/material/text_field/text_field.0.dart
Normal file
|
@ -0,0 +1,42 @@
|
|||
// Copyright 2014 The Flutter Authors. All rights reserved.
|
||||
// Use of this source code is governed by a BSD-style license that can be
|
||||
// found in the LICENSE file.
|
||||
|
||||
// Flutter code sample for TextField
|
||||
|
||||
import 'package:flutter/material.dart';
|
||||
|
||||
class ObscuredTextFieldSample extends StatelessWidget {
|
||||
const ObscuredTextFieldSample({super.key});
|
||||
|
||||
@override
|
||||
Widget build(BuildContext context) {
|
||||
return const SizedBox(
|
||||
width: 250,
|
||||
child: TextField(
|
||||
obscureText: true,
|
||||
decoration: InputDecoration(
|
||||
border: OutlineInputBorder(),
|
||||
labelText: 'Password',
|
||||
),
|
||||
),
|
||||
);
|
||||
}
|
||||
}
|
||||
|
||||
class MyApp extends StatelessWidget {
|
||||
const MyApp({super.key});
|
||||
@override
|
||||
Widget build(BuildContext context) {
|
||||
return MaterialApp(
|
||||
home: Scaffold(
|
||||
appBar: AppBar(title: const Text('Obscured Textfield')),
|
||||
body: const Center(
|
||||
child: ObscuredTextFieldSample(),
|
||||
),
|
||||
),
|
||||
);
|
||||
}
|
||||
}
|
||||
|
||||
void main() => runApp(const MyApp());
|
19
examples/api/test/material/text_field/text_field.0_test.dart
Normal file
19
examples/api/test/material/text_field/text_field.0_test.dart
Normal file
|
@ -0,0 +1,19 @@
|
|||
// Copyright 2014 The Flutter Authors. All rights reserved.
|
||||
// Use of this source code is governed by a BSD-style license that can be
|
||||
// found in the LICENSE file.
|
||||
|
||||
import 'package:flutter/material.dart';
|
||||
import 'package:flutter_api_samples/material/text_field/text_field.0.dart' as example;
|
||||
import 'package:flutter_test/flutter_test.dart';
|
||||
|
||||
void main() {
|
||||
testWidgets('TextField is obscured and has "Password" as labelText', (WidgetTester tester) async {
|
||||
await tester.pumpWidget(const example.MyApp());
|
||||
|
||||
expect(find.byType(TextField), findsOneWidget);
|
||||
|
||||
final TextField textField = tester.widget<TextField>(find.byType(TextField));
|
||||
expect(textField.obscureText, isTrue);
|
||||
expect(textField.decoration!.labelText, 'Password');
|
||||
});
|
||||
}
|
|
@ -129,22 +129,14 @@ class _TextFieldSelectionGestureDetectorBuilder extends TextSelectionGestureDete
|
|||
/// when it is no longer needed. This will ensure we discard any resources used
|
||||
/// by the object.
|
||||
///
|
||||
/// {@tool snippet}
|
||||
/// ## Obscured Input
|
||||
///
|
||||
/// {@tool dartpad}
|
||||
/// This example shows how to create a [TextField] that will obscure input. The
|
||||
/// [InputDecoration] surrounds the field in a border using [OutlineInputBorder]
|
||||
/// and adds a label.
|
||||
///
|
||||
/// 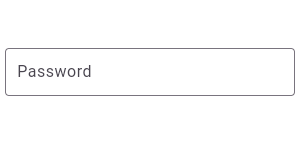
|
||||
///
|
||||
/// ```dart
|
||||
/// const TextField(
|
||||
/// obscureText: true,
|
||||
/// decoration: InputDecoration(
|
||||
/// border: OutlineInputBorder(),
|
||||
/// labelText: 'Password',
|
||||
/// ),
|
||||
/// )
|
||||
/// ```
|
||||
/// ** See code in examples/api/lib/material/text_field/text_field.0.dart **
|
||||
/// {@end-tool}
|
||||
///
|
||||
/// ## Reading values
|
||||
|
|
Loading…
Reference in a new issue