mirror of
https://github.com/flutter/flutter
synced 2024-10-13 19:52:53 +00:00
Added Dartpad and Image examples to Slider and RangeSlider docs (#57047)
This commit is contained in:
parent
5fa1c60b17
commit
a1a5781953
|
@ -31,6 +31,39 @@ typedef PaintRangeValueIndicator = void Function(PaintingContext context, Offset
|
|||
///
|
||||
/// Used to select a range from a range of values.
|
||||
///
|
||||
/// {@tool dartpad --template=stateful_widget_scaffold}
|
||||
///
|
||||
/// 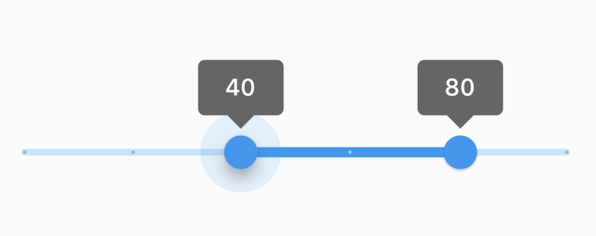
|
||||
///
|
||||
/// This range values are in intervals of 20 because the Range Slider has 5
|
||||
/// divisions, from 0 to 100. This means are values are split between 0, 20, 40,
|
||||
/// 60, 80, and 100. The range values are initialized with 40 and 80 in this demo.
|
||||
///
|
||||
/// ```dart
|
||||
/// RangeValues _currentRangeValues = const RangeValues(40, 80);
|
||||
///
|
||||
/// @override
|
||||
/// Widget build(BuildContext context) {
|
||||
/// return RangeSlider(
|
||||
/// values: _currentRangeValues,
|
||||
/// min: 0,
|
||||
/// max: 100,
|
||||
/// divisions: 5,
|
||||
/// labels: RangeLabels(
|
||||
/// _currentRangeValues.start.round().toString(),
|
||||
/// _currentRangeValues.end.round().toString(),
|
||||
/// ),
|
||||
/// onChanged: (RangeValues values) {
|
||||
/// setState(() {
|
||||
/// _currentRangeValues = values;
|
||||
/// });
|
||||
/// },
|
||||
/// );
|
||||
/// }
|
||||
/// ```
|
||||
/// {@end-tool}
|
||||
///
|
||||
/// A range slider can be used to select from either a continuous or a discrete
|
||||
/// set of values. The default is to use a continuous range of values from [min]
|
||||
/// to [max]. To use discrete values, use a non-null value for [divisions], which
|
||||
|
|
|
@ -44,6 +44,35 @@ enum _SliderType { material, adaptive }
|
|||
///
|
||||
/// Used to select from a range of values.
|
||||
///
|
||||
/// {@tool dartpad --template=stateful_widget_scaffold}
|
||||
///
|
||||
/// 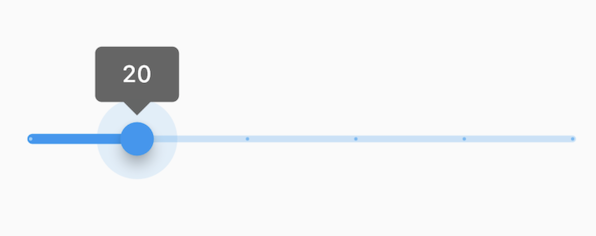
|
||||
///
|
||||
/// The Sliders value is part of the Stateful widget subclass to change the value
|
||||
/// setState was called.
|
||||
///
|
||||
/// ```dart
|
||||
/// double _currentSliderValue = 20;
|
||||
///
|
||||
/// @override
|
||||
/// Widget build(BuildContext context) {
|
||||
/// return Slider(
|
||||
/// value: _currentSliderValue,
|
||||
/// min: 0,
|
||||
/// max: 100,
|
||||
/// divisions: 5,
|
||||
/// label: _currentSliderValue.round().toString(),
|
||||
/// onChanged: (double value) {
|
||||
/// setState(() {
|
||||
/// _currentSliderValue = value;
|
||||
/// });
|
||||
/// },
|
||||
/// );
|
||||
/// }
|
||||
/// ```
|
||||
/// {@end-tool}
|
||||
///
|
||||
/// A slider can be used to select from either a continuous or a discrete set of
|
||||
/// values. The default is to use a continuous range of values from [min] to
|
||||
/// [max]. To use discrete values, use a non-null value for [divisions], which
|
||||
|
|
|
@ -1556,6 +1556,9 @@ abstract class BaseSliderTrackShape {
|
|||
///
|
||||
/// {@macro flutter.material.slider.trackSegment}
|
||||
///
|
||||
/// ![A slider widget, consisting of 5 divisions and showing the rectangular slider track shape.]
|
||||
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rectangular_slider_track_shape.png)
|
||||
///
|
||||
/// See also:
|
||||
///
|
||||
/// * [Slider], for the component that is meant to display this shape.
|
||||
|
@ -1661,6 +1664,9 @@ class RectangularSliderTrackShape extends SliderTrackShape with BaseSliderTrackS
|
|||
///
|
||||
/// {@macro flutter.material.slider.trackSegment}
|
||||
///
|
||||
/// ![A slider widget, consisting of 5 divisions and showing the rounded rect slider track shape.]
|
||||
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rounded_rect_slider_track_shape.png)
|
||||
///
|
||||
/// See also:
|
||||
///
|
||||
/// * [Slider], for the component that is meant to display this shape.
|
||||
|
@ -1773,6 +1779,9 @@ class RoundedRectSliderTrackShape extends SliderTrackShape with BaseSliderTrackS
|
|||
///
|
||||
/// {@macro flutter.material.rangeSlider.trackSegment}
|
||||
///
|
||||
/// ![A range slider widget, consisting of 5 divisions and showing the rectangular range slider track shape.]
|
||||
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rectangular_range_slider_track_shape.png)
|
||||
///
|
||||
/// See also:
|
||||
///
|
||||
/// * [RangeSlider], for the component that is meant to display this shape.
|
||||
|
@ -1900,6 +1909,9 @@ class RectangularRangeSliderTrackShape extends RangeSliderTrackShape {
|
|||
///
|
||||
/// {@macro flutter.material.rangeSlider.trackSegment}
|
||||
///
|
||||
/// ![A range slider widget, consisting of 5 divisions and showing the rounded rect range slider track shape.]
|
||||
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rounded_rect_range_slider_track_shape.png)
|
||||
///
|
||||
/// See also:
|
||||
///
|
||||
/// * [RangeSlider], for the component that is meant to display this shape.
|
||||
|
@ -2062,6 +2074,9 @@ class RoundedRectRangeSliderTrackShape extends RangeSliderTrackShape {
|
|||
/// [SliderThemeData.disabledActiveTrackColor],
|
||||
/// [SliderThemeData.disabledInactiveTrackColor].
|
||||
///
|
||||
/// ![A slider widget, consisting of 5 divisions and showing the round slider slider tick mark shape.]
|
||||
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rounded_slider_tick_mark_shape.png)
|
||||
///
|
||||
/// See also:
|
||||
///
|
||||
/// * [Slider], which includes tick marks defined by this shape.
|
||||
|
@ -2157,6 +2172,9 @@ class RoundSliderTickMarkShape extends SliderTickMarkShape {
|
|||
/// [SliderThemeData.disabledActiveTrackColor],
|
||||
/// [SliderThemeData.disabledInactiveTrackColor].
|
||||
///
|
||||
/// ![A slider widget, consisting of 5 divisions and showing the round range slider tick mark shape.]
|
||||
/// (https://flutter.github.io/assets-for-api-docs/assets/material/round_range_slider_tick_mark_shape.png )
|
||||
///
|
||||
/// See also:
|
||||
///
|
||||
/// * [RangeSlider], which includes tick marks defined by this shape.
|
||||
|
@ -2301,6 +2319,9 @@ class _EmptySliderComponentShape extends SliderComponentShape {
|
|||
///
|
||||
/// There is a shadow for the resting, pressed, hovered, and focused state.
|
||||
///
|
||||
/// ![A slider widget, consisting of 5 divisions and showing the round slider thumb shape.]
|
||||
/// (https://flutter.github.io/assets-for-api-docs/assets/material/round_slider_thumb_shape.png)
|
||||
///
|
||||
/// See also:
|
||||
///
|
||||
/// * [Slider], which includes a thumb defined by this shape.
|
||||
|
@ -2407,6 +2428,9 @@ class RoundSliderThumbShape extends SliderComponentShape {
|
|||
///
|
||||
/// There is a shadow for the resting and pressed state.
|
||||
///
|
||||
/// ![A slider widget, consisting of 5 divisions and showing the round range slider thumb shape.]
|
||||
/// (https://flutter.github.io/assets-for-api-docs/assets/material/round_range_slider_thumb_shape.png)
|
||||
///
|
||||
/// See also:
|
||||
///
|
||||
/// * [RangeSlider], which includes thumbs defined by this shape.
|
||||
|
@ -2598,6 +2622,9 @@ class RoundSliderOverlayShape extends SliderComponentShape {
|
|||
|
||||
/// The default shape of a [Slider]'s value indicator.
|
||||
///
|
||||
/// ![A slider widget, consisting of 5 divisions and showing the rectangular slider value indicator shape.]
|
||||
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rectangular_slider_value_indicator_shape.png)
|
||||
///
|
||||
/// See also:
|
||||
///
|
||||
/// * [Slider], which includes a value indicator defined by this shape.
|
||||
|
@ -2652,6 +2679,9 @@ class RectangularSliderValueIndicatorShape extends SliderComponentShape {
|
|||
|
||||
/// The default shape of a [RangeSlider]'s value indicators.
|
||||
///
|
||||
/// ![A slider widget, consisting of 5 divisions and showing the rectangular range slider value indicator shape.]
|
||||
/// (https://flutter.github.io/assets-for-api-docs/assets/material/rectangular_range_slider_value_indicator_shape.png)
|
||||
///
|
||||
/// See also:
|
||||
///
|
||||
/// * [RangeSlider], which includes value indicators defined by this shape.
|
||||
|
@ -2856,6 +2886,9 @@ class _RectangularSliderValueIndicatorPathPainter {
|
|||
/// A variant shape of a [Slider]'s value indicator . The value indicator is in
|
||||
/// the shape of an upside-down pear.
|
||||
///
|
||||
/// ![A slider widget, consisting of 5 divisions and showing the paddle slider value indicator shape.]
|
||||
/// (https://flutter.github.io/assets-for-api-docs/assets/material/paddle_slider_value_indicator_shape.png)
|
||||
///
|
||||
/// See also:
|
||||
///
|
||||
/// * [Slider], which includes a value indicator defined by this shape.
|
||||
|
@ -2918,6 +2951,9 @@ class PaddleSliderValueIndicatorShape extends SliderComponentShape {
|
|||
/// A variant shape of a [RangeSlider]'s value indicators. The value indicator
|
||||
/// is in the shape of an upside-down pear.
|
||||
///
|
||||
/// ![A slider widget, consisting of 5 divisions and showing the paddle range slider value indicator shape.]
|
||||
/// (https://flutter.github.io/assets-for-api-docs/assets/material/paddle_range_slider_value_indicator_shape.png)
|
||||
///
|
||||
/// See also:
|
||||
///
|
||||
/// * [RangeSlider], which includes value indicators defined by this shape.
|
||||
|
|
Loading…
Reference in a new issue