mirror of
https://github.com/denoland/deno
synced 2024-09-13 21:31:18 +00:00
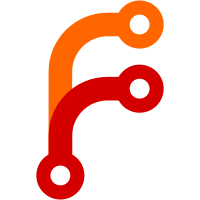
This PR: 1. Replaces `@test_util/std`-prefixed imports with `@std`. 2. Adds `@std/` import map entries to a few `deno.json` files.
66 lines
2.2 KiB
TypeScript
66 lines
2.2 KiB
TypeScript
// Copyright 2018-2024 the Deno authors. All rights reserved. MIT license.
|
|
import { assert, assertEquals } from "./test_util.ts";
|
|
import { assertType, IsExact } from "@std/testing/types.ts";
|
|
|
|
Deno.test(function urlPatternFromString() {
|
|
const pattern = new URLPattern("https://deno.land/foo/:bar");
|
|
assertEquals(pattern.protocol, "https");
|
|
assertEquals(pattern.hostname, "deno.land");
|
|
assertEquals(pattern.pathname, "/foo/:bar");
|
|
|
|
assert(pattern.test("https://deno.land/foo/x"));
|
|
assert(!pattern.test("https://deno.com/foo/x"));
|
|
const match = pattern.exec("https://deno.land/foo/x");
|
|
assert(match);
|
|
assertEquals(match.pathname.input, "/foo/x");
|
|
assertEquals(match.pathname.groups, { bar: "x" });
|
|
|
|
// group values should be nullable
|
|
const val = match.pathname.groups.val;
|
|
assertType<IsExact<typeof val, string | undefined>>(true);
|
|
});
|
|
|
|
Deno.test(function urlPatternFromStringWithBase() {
|
|
const pattern = new URLPattern("/foo/:bar", "https://deno.land");
|
|
assertEquals(pattern.protocol, "https");
|
|
assertEquals(pattern.hostname, "deno.land");
|
|
assertEquals(pattern.pathname, "/foo/:bar");
|
|
|
|
assert(pattern.test("https://deno.land/foo/x"));
|
|
assert(!pattern.test("https://deno.com/foo/x"));
|
|
const match = pattern.exec("https://deno.land/foo/x");
|
|
assert(match);
|
|
assertEquals(match.pathname.input, "/foo/x");
|
|
assertEquals(match.pathname.groups, { bar: "x" });
|
|
});
|
|
|
|
Deno.test(function urlPatternFromInit() {
|
|
const pattern = new URLPattern({
|
|
pathname: "/foo/:bar",
|
|
});
|
|
assertEquals(pattern.protocol, "*");
|
|
assertEquals(pattern.hostname, "*");
|
|
assertEquals(pattern.pathname, "/foo/:bar");
|
|
|
|
assert(pattern.test("https://deno.land/foo/x"));
|
|
assert(pattern.test("https://deno.com/foo/x"));
|
|
assert(!pattern.test("https://deno.com/bar/x"));
|
|
|
|
assert(pattern.test({ pathname: "/foo/x" }));
|
|
});
|
|
|
|
Deno.test(function urlPatternWithPrototypePollution() {
|
|
const originalExec = RegExp.prototype.exec;
|
|
try {
|
|
RegExp.prototype.exec = () => {
|
|
throw Error();
|
|
};
|
|
const pattern = new URLPattern({
|
|
pathname: "/foo/:bar",
|
|
});
|
|
assert(pattern.test("https://deno.land/foo/x"));
|
|
} finally {
|
|
RegExp.prototype.exec = originalExec;
|
|
}
|
|
});
|