mirror of
https://github.com/denoland/deno
synced 2024-08-27 03:50:16 +00:00
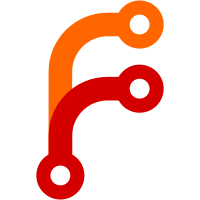
Code run within Deno-mode and Node-mode should have access to a slightly different set of globals. Previously this was done through a compile time code-transform for Node-mode, but this is not ideal and has many edge cases, for example Node's globalThis having a different identity than Deno's globalThis. This commit makes the `globalThis` of the entire runtime a semi-proxy. This proxy returns a different set of globals depending on the caller's mode. This is not a full proxy, because it is shadowed by "real" properties on globalThis. This is done to avoid the overhead of a full proxy for all globalThis operations. The globals between Deno-mode and Node-mode are now properly segregated. This means that code running in Deno-mode will not have access to Node's globals, and vice versa. Deleting a managed global in Deno-mode will NOT delete the corresponding global in Node-mode, and vice versa. --------- Co-authored-by: Bartek Iwańczuk <biwanczuk@gmail.com> Co-authored-by: Aapo Alasuutari <aapo.alasuutari@gmail.com>
36 lines
795 B
Rust
36 lines
795 B
Rust
// Copyright 2018-2023 the Deno authors. All rights reserved. MIT license.
|
|
|
|
use deno_core::Snapshot;
|
|
use log::debug;
|
|
|
|
static CLI_SNAPSHOT: &[u8] =
|
|
include_bytes!(concat!(env!("OUT_DIR"), "/CLI_SNAPSHOT.bin"));
|
|
|
|
pub fn deno_isolate_init() -> Snapshot {
|
|
debug!("Deno isolate init with snapshots.");
|
|
Snapshot::Static(CLI_SNAPSHOT)
|
|
}
|
|
|
|
#[cfg(test)]
|
|
mod tests {
|
|
use super::*;
|
|
|
|
#[test]
|
|
fn runtime_snapshot() {
|
|
let mut js_runtime = deno_core::JsRuntime::new(deno_core::RuntimeOptions {
|
|
startup_snapshot: Some(deno_isolate_init()),
|
|
..Default::default()
|
|
});
|
|
js_runtime
|
|
.execute_script_static(
|
|
"<anon>",
|
|
r#"
|
|
if (!(bootstrap.mainRuntime && bootstrap.workerRuntime)) {
|
|
throw Error("bad");
|
|
}
|
|
"#,
|
|
)
|
|
.unwrap();
|
|
}
|
|
}
|