mirror of
https://github.com/denoland/deno
synced 2024-10-13 19:42:29 +00:00
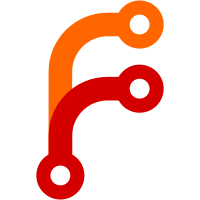
This commit fixes panic occurring if $DENO_DIR is set to a relative path, eg. "DENO_DIR=denodir deno run main.ts". Before creating DenoDir instance given path is checked and if necessary resolved against current working directory. Additional sanity checks were put in place to ensure all caches receive absolute path for the location.
48 lines
1.4 KiB
Rust
48 lines
1.4 KiB
Rust
// Copyright 2018-2020 the Deno authors. All rights reserved. MIT license.
|
|
use crate::disk_cache::DiskCache;
|
|
use std::path::PathBuf;
|
|
|
|
/// `DenoDir` serves as coordinator for multiple `DiskCache`s containing them
|
|
/// in single directory that can be controlled with `$DENO_DIR` env variable.
|
|
#[derive(Clone)]
|
|
pub struct DenoDir {
|
|
// Example: /Users/rld/.deno/
|
|
pub root: PathBuf,
|
|
/// Used by TsCompiler to cache compiler output.
|
|
pub gen_cache: DiskCache,
|
|
}
|
|
|
|
impl DenoDir {
|
|
pub fn new(maybe_custom_root: Option<PathBuf>) -> std::io::Result<Self> {
|
|
// Only setup once.
|
|
let home_dir = dirs::home_dir().expect("Could not get home directory.");
|
|
let fallback = home_dir.join(".deno");
|
|
// We use the OS cache dir because all files deno writes are cache files
|
|
// Once that changes we need to start using different roots if DENO_DIR
|
|
// is not set, and keep a single one if it is.
|
|
let default = dirs::cache_dir()
|
|
.map(|d| d.join("deno"))
|
|
.unwrap_or(fallback);
|
|
|
|
let root: PathBuf = if let Some(root) = maybe_custom_root {
|
|
if root.is_absolute() {
|
|
root
|
|
} else {
|
|
std::env::current_dir()?.join(root)
|
|
}
|
|
} else {
|
|
default
|
|
};
|
|
assert!(root.is_absolute());
|
|
let gen_path = root.join("gen");
|
|
|
|
let deno_dir = Self {
|
|
root,
|
|
gen_cache: DiskCache::new(&gen_path),
|
|
};
|
|
deno_dir.gen_cache.ensure_location()?;
|
|
|
|
Ok(deno_dir)
|
|
}
|
|
}
|