mirror of
https://github.com/denoland/deno
synced 2024-11-05 18:45:24 +00:00
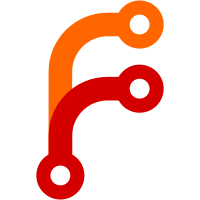
Updating categories for new sitemap as documented here: https://lucid.app/lucidspark/744b0498-a133-494d-981c-76059dd18885/edit?view_items=jpvBwFdYlNdB&invitationId=inv_50c83415-2aa5-423f-b438-ea156695c08b
59 lines
1.4 KiB
TypeScript
59 lines
1.4 KiB
TypeScript
// Copyright 2018-2024 the Deno authors. All rights reserved. MIT license.
|
|
|
|
// deno-lint-ignore-file no-var
|
|
|
|
/// <reference no-default-lib="true" />
|
|
/// <reference lib="esnext" />
|
|
|
|
/** @category Canvas */
|
|
declare type ColorSpaceConversion = "default" | "none";
|
|
|
|
/** @category Canvas */
|
|
declare type ImageOrientation = "flipY" | "from-image" | "none";
|
|
|
|
/** @category Canvas */
|
|
declare type PremultiplyAlpha = "default" | "none" | "premultiply";
|
|
|
|
/** @category Canvas */
|
|
declare type ResizeQuality = "high" | "low" | "medium" | "pixelated";
|
|
|
|
/** @category Canvas */
|
|
declare type ImageBitmapSource = Blob | ImageData;
|
|
|
|
/** @category Canvas */
|
|
declare interface ImageBitmapOptions {
|
|
colorSpaceConversion?: ColorSpaceConversion;
|
|
imageOrientation?: ImageOrientation;
|
|
premultiplyAlpha?: PremultiplyAlpha;
|
|
resizeHeight?: number;
|
|
resizeQuality?: ResizeQuality;
|
|
resizeWidth?: number;
|
|
}
|
|
|
|
/** @category Canvas */
|
|
declare function createImageBitmap(
|
|
image: ImageBitmapSource,
|
|
options?: ImageBitmapOptions,
|
|
): Promise<ImageBitmap>;
|
|
/** @category Canvas */
|
|
declare function createImageBitmap(
|
|
image: ImageBitmapSource,
|
|
sx: number,
|
|
sy: number,
|
|
sw: number,
|
|
sh: number,
|
|
options?: ImageBitmapOptions,
|
|
): Promise<ImageBitmap>;
|
|
|
|
/** @category Canvas */
|
|
declare interface ImageBitmap {
|
|
readonly height: number;
|
|
readonly width: number;
|
|
close(): void;
|
|
}
|
|
|
|
/** @category Canvas */
|
|
declare var ImageBitmap: {
|
|
prototype: ImageBitmap;
|
|
new (): ImageBitmap;
|
|
};
|