mirror of
https://github.com/denoland/deno
synced 2024-09-13 21:31:18 +00:00
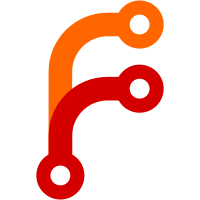
This looks like a massive PR, but it's only a move from cli/tests -> tests, and updates of relative paths for files. This is the first step towards aggregate all of the integration test files under tests/, which will lead to a set of integration tests that can run without the CLI binary being built. While we could leave these tests under `cli`, it would require us to keep a more complex directory structure for the various test runners. In addition, we have a lot of complexity to ignore various test files in the `cli` project itself (cargo publish exclusion rules, autotests = false, etc). And finally, the `tests/` folder will eventually house the `test_ffi`, `test_napi` and other testing code, reducing the size of the root repo directory. For easier review, the extremely large and noisy "move" is in the first commit (with no changes -- just a move), while the remainder of the changes to actual files is in the second commit.
226 lines
6 KiB
TypeScript
226 lines
6 KiB
TypeScript
// Copyright 2018-2024 the Deno authors. All rights reserved. MIT license.
|
|
// deno-lint-ignore-file no-window-prefix
|
|
import { assert, assertEquals, assertRejects } from "./test_util.ts";
|
|
|
|
Deno.test(function globalThisExists() {
|
|
assert(globalThis != null);
|
|
});
|
|
|
|
Deno.test(function noInternalGlobals() {
|
|
// globalThis.__bootstrap should not be there.
|
|
for (const key of Object.keys(globalThis)) {
|
|
assert(!key.startsWith("_"));
|
|
}
|
|
});
|
|
|
|
Deno.test(function windowExists() {
|
|
assert(window != null);
|
|
});
|
|
|
|
Deno.test(function selfExists() {
|
|
assert(self != null);
|
|
});
|
|
|
|
Deno.test(function windowWindowExists() {
|
|
assert(window.window === window);
|
|
});
|
|
|
|
Deno.test(function windowSelfExists() {
|
|
assert(window.self === window);
|
|
});
|
|
|
|
Deno.test(function globalThisEqualsWindow() {
|
|
assert(globalThis === window);
|
|
});
|
|
|
|
Deno.test(function globalThisEqualsSelf() {
|
|
assert(globalThis === self);
|
|
});
|
|
|
|
Deno.test(function globalThisInstanceofWindow() {
|
|
assert(globalThis instanceof Window);
|
|
});
|
|
|
|
Deno.test(function globalThisConstructorLength() {
|
|
assert(globalThis.constructor.length === 0);
|
|
});
|
|
|
|
Deno.test(function globalThisInstanceofEventTarget() {
|
|
assert(globalThis instanceof EventTarget);
|
|
});
|
|
|
|
Deno.test(function navigatorInstanceofNavigator() {
|
|
// TODO(nayeemrmn): Add `Navigator` to deno_lint globals.
|
|
// deno-lint-ignore no-undef
|
|
assert(navigator instanceof Navigator);
|
|
});
|
|
|
|
Deno.test(function DenoNamespaceExists() {
|
|
assert(Deno != null);
|
|
});
|
|
|
|
Deno.test(function DenoNamespaceEqualsWindowDeno() {
|
|
assert(Deno === window.Deno);
|
|
});
|
|
|
|
Deno.test(function DenoNamespaceIsNotFrozen() {
|
|
assert(!Object.isFrozen(Deno));
|
|
});
|
|
|
|
Deno.test(function webAssemblyExists() {
|
|
assert(typeof WebAssembly.compile === "function");
|
|
});
|
|
|
|
// @ts-ignore This is not publicly typed namespace, but it's there for sure.
|
|
const core = Deno[Deno.internal].core;
|
|
|
|
Deno.test(function DenoNamespaceConfigurable() {
|
|
const desc = Object.getOwnPropertyDescriptor(globalThis, "Deno");
|
|
assert(desc);
|
|
assert(desc.configurable);
|
|
assert(!desc.writable);
|
|
});
|
|
|
|
Deno.test(function DenoCoreNamespaceIsImmutable() {
|
|
const { print } = core;
|
|
try {
|
|
core.print = 1;
|
|
} catch {
|
|
// pass
|
|
}
|
|
assert(print === core.print);
|
|
try {
|
|
delete core.print;
|
|
} catch {
|
|
// pass
|
|
}
|
|
assert(print === core.print);
|
|
});
|
|
|
|
Deno.test(async function windowQueueMicrotask() {
|
|
let resolve1: () => void | undefined;
|
|
let resolve2: () => void | undefined;
|
|
let microtaskDone = false;
|
|
const p1 = new Promise<void>((res) => {
|
|
resolve1 = () => {
|
|
microtaskDone = true;
|
|
res();
|
|
};
|
|
});
|
|
const p2 = new Promise<void>((res) => {
|
|
resolve2 = () => {
|
|
assert(microtaskDone);
|
|
res();
|
|
};
|
|
});
|
|
window.queueMicrotask(resolve1!);
|
|
setTimeout(resolve2!, 0);
|
|
await p1;
|
|
await p2;
|
|
});
|
|
|
|
Deno.test(function webApiGlobalThis() {
|
|
assert(globalThis.FormData !== null);
|
|
assert(globalThis.TextEncoder !== null);
|
|
assert(globalThis.TextEncoderStream !== null);
|
|
assert(globalThis.TextDecoder !== null);
|
|
assert(globalThis.TextDecoderStream !== null);
|
|
assert(globalThis.CountQueuingStrategy !== null);
|
|
assert(globalThis.ByteLengthQueuingStrategy !== null);
|
|
});
|
|
|
|
Deno.test(function windowNameIsDefined() {
|
|
assertEquals(typeof globalThis.name, "string");
|
|
assertEquals(name, "");
|
|
assertEquals(window.name, name);
|
|
name = "foobar";
|
|
assertEquals(window.name, "foobar");
|
|
assertEquals(name, "foobar");
|
|
name = "";
|
|
assertEquals(window.name, "");
|
|
assertEquals(name, "");
|
|
});
|
|
|
|
Deno.test(async function promiseWithResolvers() {
|
|
{
|
|
const { promise, resolve } = Promise.withResolvers();
|
|
resolve(true);
|
|
assert(await promise);
|
|
}
|
|
{
|
|
const { promise, reject } = Promise.withResolvers();
|
|
reject(new Error("boom!"));
|
|
await assertRejects(() => promise, Error, "boom!");
|
|
}
|
|
});
|
|
|
|
Deno.test(async function arrayFromAsync() {
|
|
// Taken from https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/fromAsync#examples
|
|
// Thank you.
|
|
const asyncIterable = (async function* () {
|
|
for (let i = 0; i < 5; i++) {
|
|
await new Promise((resolve) => setTimeout(resolve, 10 * i));
|
|
yield i;
|
|
}
|
|
})();
|
|
|
|
const a = await Array.fromAsync(asyncIterable);
|
|
assertEquals(a, [0, 1, 2, 3, 4]);
|
|
|
|
const b = await Array.fromAsync(new Map([[1, 2], [3, 4]]));
|
|
assertEquals(b, [[1, 2], [3, 4]]);
|
|
});
|
|
|
|
// Taken from https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/groupBy#examples
|
|
Deno.test(function objectGroupBy() {
|
|
const inventory = [
|
|
{ name: "asparagus", type: "vegetables", quantity: 5 },
|
|
{ name: "bananas", type: "fruit", quantity: 0 },
|
|
{ name: "goat", type: "meat", quantity: 23 },
|
|
{ name: "cherries", type: "fruit", quantity: 5 },
|
|
{ name: "fish", type: "meat", quantity: 22 },
|
|
];
|
|
const result = Object.groupBy(inventory, ({ type }) => type);
|
|
assertEquals(result, {
|
|
vegetables: [
|
|
{ name: "asparagus", type: "vegetables", quantity: 5 },
|
|
],
|
|
fruit: [
|
|
{ name: "bananas", type: "fruit", quantity: 0 },
|
|
{ name: "cherries", type: "fruit", quantity: 5 },
|
|
],
|
|
meat: [
|
|
{ name: "goat", type: "meat", quantity: 23 },
|
|
{ name: "fish", type: "meat", quantity: 22 },
|
|
],
|
|
});
|
|
});
|
|
|
|
Deno.test(function objectGroupByEmpty() {
|
|
const empty: string[] = [];
|
|
const result = Object.groupBy(empty, () => "abc");
|
|
assertEquals(result.abc, undefined);
|
|
});
|
|
|
|
// Taken from https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Map/groupBy#examples
|
|
Deno.test(function mapGroupBy() {
|
|
const inventory = [
|
|
{ name: "asparagus", type: "vegetables", quantity: 9 },
|
|
{ name: "bananas", type: "fruit", quantity: 5 },
|
|
{ name: "goat", type: "meat", quantity: 23 },
|
|
{ name: "cherries", type: "fruit", quantity: 12 },
|
|
{ name: "fish", type: "meat", quantity: 22 },
|
|
];
|
|
const restock = { restock: true };
|
|
const sufficient = { restock: false };
|
|
const result = Map.groupBy(
|
|
inventory,
|
|
({ quantity }) => quantity < 6 ? restock : sufficient,
|
|
);
|
|
assertEquals(result.get(restock), [{
|
|
name: "bananas",
|
|
type: "fruit",
|
|
quantity: 5,
|
|
}]);
|
|
});
|