mirror of
https://github.com/denoland/deno
synced 2024-11-05 18:45:24 +00:00
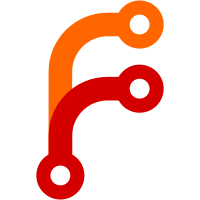
This PR adds unstable `Deno.cron` API to trigger execution of cron jobs. * State: All cron state is in memory. Cron jobs are scheduled according to the cron schedule expression and the current time. No state is persisted to disk. * Time zone: Cron expressions specify time in UTC. * Overlapping executions: not permitted. If the next scheduled execution time occurs while the same cron job is still executing, the scheduled execution is skipped. * Retries: failed jobs are automatically retried until they succeed or until retry threshold is reached. Retry policy can be optionally specified using `options.backoffSchedule`.
58 lines
1.3 KiB
TypeScript
58 lines
1.3 KiB
TypeScript
// Copyright 2018-2023 the Deno authors. All rights reserved. MIT license.
|
|
|
|
// @ts-ignore internal api
|
|
const core = Deno.core;
|
|
|
|
function cron(
|
|
name: string,
|
|
schedule: string,
|
|
handler: () => Promise<void> | void,
|
|
options?: { backoffSchedule?: number[]; signal?: AbortSignal },
|
|
) {
|
|
if (name === undefined) {
|
|
throw new TypeError("Deno.cron requires a unique name");
|
|
}
|
|
if (schedule === undefined) {
|
|
throw new TypeError("Deno.cron requires a valid schedule");
|
|
}
|
|
if (handler === undefined) {
|
|
throw new TypeError("Deno.cron requires a handler");
|
|
}
|
|
|
|
const rid = core.ops.op_cron_create(
|
|
name,
|
|
schedule,
|
|
options?.backoffSchedule,
|
|
);
|
|
|
|
if (options?.signal) {
|
|
const signal = options?.signal;
|
|
signal.addEventListener(
|
|
"abort",
|
|
() => {
|
|
core.close(rid);
|
|
},
|
|
{ once: true },
|
|
);
|
|
}
|
|
|
|
return (async () => {
|
|
let success = true;
|
|
while (true) {
|
|
const r = await core.opAsync("op_cron_next", rid, success);
|
|
if (r === false) {
|
|
break;
|
|
}
|
|
try {
|
|
const result = handler();
|
|
const _res = result instanceof Promise ? (await result) : result;
|
|
success = true;
|
|
} catch (error) {
|
|
console.error(`Exception in cron handler ${name}`, error);
|
|
success = false;
|
|
}
|
|
}
|
|
})();
|
|
}
|
|
|
|
export { cron };
|