mirror of
https://github.com/denoland/deno
synced 2024-09-13 05:11:43 +00:00
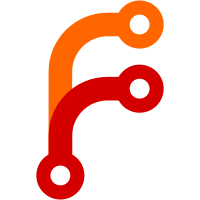
This looks like a massive PR, but it's only a move from cli/tests -> tests, and updates of relative paths for files. This is the first step towards aggregate all of the integration test files under tests/, which will lead to a set of integration tests that can run without the CLI binary being built. While we could leave these tests under `cli`, it would require us to keep a more complex directory structure for the various test runners. In addition, we have a lot of complexity to ignore various test files in the `cli` project itself (cargo publish exclusion rules, autotests = false, etc). And finally, the `tests/` folder will eventually house the `test_ffi`, `test_napi` and other testing code, reducing the size of the root repo directory. For easier review, the extremely large and noisy "move" is in the first commit (with no changes -- just a move), while the remainder of the changes to actual files is in the second commit.
103 lines
3.1 KiB
TypeScript
103 lines
3.1 KiB
TypeScript
// Copyright 2018-2024 the Deno authors. All rights reserved. MIT license.
|
|
import {
|
|
assert,
|
|
assertEquals,
|
|
assertStringIncludes,
|
|
assertThrows,
|
|
} from "./test_util.ts";
|
|
|
|
Deno.test(async function responseText() {
|
|
const response = new Response("hello world");
|
|
const textPromise = response.text();
|
|
assert(textPromise instanceof Promise);
|
|
const text = await textPromise;
|
|
assert(typeof text === "string");
|
|
assertEquals(text, "hello world");
|
|
});
|
|
|
|
Deno.test(async function responseArrayBuffer() {
|
|
const response = new Response(new Uint8Array([1, 2, 3]));
|
|
const arrayBufferPromise = response.arrayBuffer();
|
|
assert(arrayBufferPromise instanceof Promise);
|
|
const arrayBuffer = await arrayBufferPromise;
|
|
assert(arrayBuffer instanceof ArrayBuffer);
|
|
assertEquals(new Uint8Array(arrayBuffer), new Uint8Array([1, 2, 3]));
|
|
});
|
|
|
|
Deno.test(async function responseJson() {
|
|
const response = new Response('{"hello": "world"}');
|
|
const jsonPromise = response.json();
|
|
assert(jsonPromise instanceof Promise);
|
|
const json = await jsonPromise;
|
|
assert(json instanceof Object);
|
|
assertEquals(json, { hello: "world" });
|
|
});
|
|
|
|
Deno.test(async function responseBlob() {
|
|
const response = new Response(new Uint8Array([1, 2, 3]));
|
|
const blobPromise = response.blob();
|
|
assert(blobPromise instanceof Promise);
|
|
const blob = await blobPromise;
|
|
assert(blob instanceof Blob);
|
|
assertEquals(blob.size, 3);
|
|
assertEquals(await blob.arrayBuffer(), new Uint8Array([1, 2, 3]).buffer);
|
|
});
|
|
|
|
Deno.test(async function responseFormData() {
|
|
const input = new FormData();
|
|
input.append("hello", "world");
|
|
const response = new Response(input);
|
|
const contentType = response.headers.get("content-type")!;
|
|
assert(contentType.startsWith("multipart/form-data"));
|
|
const formDataPromise = response.formData();
|
|
assert(formDataPromise instanceof Promise);
|
|
const formData = await formDataPromise;
|
|
assert(formData instanceof FormData);
|
|
assertEquals([...formData], [...input]);
|
|
});
|
|
|
|
Deno.test(function responseInvalidInit() {
|
|
// deno-lint-ignore ban-ts-comment
|
|
// @ts-expect-error
|
|
assertThrows(() => new Response("", 0));
|
|
assertThrows(() => new Response("", { status: 0 }));
|
|
// deno-lint-ignore ban-ts-comment
|
|
// @ts-expect-error
|
|
assertThrows(() => new Response("", { status: null }));
|
|
});
|
|
|
|
Deno.test(function responseNullInit() {
|
|
// deno-lint-ignore ban-ts-comment
|
|
// @ts-expect-error
|
|
const response = new Response("", null);
|
|
assertEquals(response.status, 200);
|
|
});
|
|
|
|
Deno.test(function customInspectFunction() {
|
|
const response = new Response();
|
|
assertEquals(
|
|
Deno.inspect(response),
|
|
`Response {
|
|
body: null,
|
|
bodyUsed: false,
|
|
headers: Headers {},
|
|
ok: true,
|
|
redirected: false,
|
|
status: 200,
|
|
statusText: "",
|
|
url: ""
|
|
}`,
|
|
);
|
|
assertStringIncludes(Deno.inspect(Response.prototype), "Response");
|
|
});
|
|
|
|
Deno.test(async function responseBodyUsed() {
|
|
const response = new Response("body");
|
|
assert(!response.bodyUsed);
|
|
await response.text();
|
|
assert(response.bodyUsed);
|
|
// .body getter is needed so we can test the faulty code path
|
|
response.body;
|
|
assert(response.bodyUsed);
|
|
});
|