mirror of
https://github.com/denoland/deno
synced 2024-09-13 05:11:43 +00:00
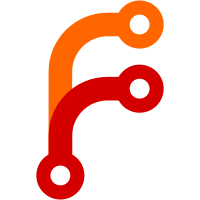
This looks like a massive PR, but it's only a move from cli/tests -> tests, and updates of relative paths for files. This is the first step towards aggregate all of the integration test files under tests/, which will lead to a set of integration tests that can run without the CLI binary being built. While we could leave these tests under `cli`, it would require us to keep a more complex directory structure for the various test runners. In addition, we have a lot of complexity to ignore various test files in the `cli` project itself (cargo publish exclusion rules, autotests = false, etc). And finally, the `tests/` folder will eventually house the `test_ffi`, `test_napi` and other testing code, reducing the size of the root repo directory. For easier review, the extremely large and noisy "move" is in the first commit (with no changes -- just a move), while the remainder of the changes to actual files is in the second commit.
55 lines
1.4 KiB
TypeScript
55 lines
1.4 KiB
TypeScript
// Copyright 2018-2024 the Deno authors. All rights reserved. MIT license.
|
|
import { assertEquals, assertMatch } from "./test_util.ts";
|
|
|
|
Deno.test(function errorStackMessageLine() {
|
|
const e1 = new Error();
|
|
e1.name = "Foo";
|
|
e1.message = "bar";
|
|
assertMatch(e1.stack!, /^Foo: bar\n/);
|
|
|
|
const e2 = new Error();
|
|
e2.name = "";
|
|
e2.message = "bar";
|
|
assertMatch(e2.stack!, /^bar\n/);
|
|
|
|
const e3 = new Error();
|
|
e3.name = "Foo";
|
|
e3.message = "";
|
|
assertMatch(e3.stack!, /^Foo\n/);
|
|
|
|
const e4 = new Error();
|
|
e4.name = "";
|
|
e4.message = "";
|
|
assertMatch(e4.stack!, /^\n/);
|
|
|
|
const e5 = new Error();
|
|
// deno-lint-ignore ban-ts-comment
|
|
// @ts-expect-error
|
|
e5.name = undefined;
|
|
// deno-lint-ignore ban-ts-comment
|
|
// @ts-expect-error
|
|
e5.message = undefined;
|
|
assertMatch(e5.stack!, /^Error\n/);
|
|
|
|
const e6 = new Error();
|
|
// deno-lint-ignore ban-ts-comment
|
|
// @ts-expect-error
|
|
e6.name = null;
|
|
// deno-lint-ignore ban-ts-comment
|
|
// @ts-expect-error
|
|
e6.message = null;
|
|
assertMatch(e6.stack!, /^null: null\n/);
|
|
});
|
|
|
|
Deno.test(function captureStackTrace() {
|
|
function foo() {
|
|
const error = new Error();
|
|
const stack1 = error.stack!;
|
|
Error.captureStackTrace(error, foo);
|
|
const stack2 = error.stack!;
|
|
// stack2 should be stack1 without the first frame.
|
|
assertEquals(stack2, stack1.replace(/(?<=^[^\n]*\n)[^\n]*\n/, ""));
|
|
}
|
|
foo();
|
|
});
|