mirror of
https://github.com/denoland/deno
synced 2024-08-25 02:57:22 +00:00
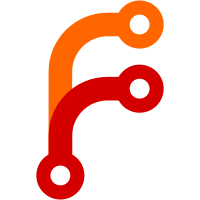
This PR: 1. Replaces `@test_util/std`-prefixed imports with `@std`. 2. Adds `@std/` import map entries to a few `deno.json` files.
52 lines
1.5 KiB
TypeScript
52 lines
1.5 KiB
TypeScript
// Copyright 2018-2024 the Deno authors. All rights reserved. MIT license.
|
|
import { write, writeSync } from "node:fs";
|
|
import { assertEquals } from "@std/assert/mod.ts";
|
|
import { Buffer } from "node:buffer";
|
|
|
|
const decoder = new TextDecoder("utf-8");
|
|
|
|
Deno.test({
|
|
name: "Data is written to the file with the correct length",
|
|
async fn() {
|
|
const tempFile: string = await Deno.makeTempFile();
|
|
using file = await Deno.open(tempFile, {
|
|
create: true,
|
|
write: true,
|
|
read: true,
|
|
});
|
|
const buffer = Buffer.from("hello world");
|
|
const bytesWrite = await new Promise((resolve, reject) => {
|
|
write(file.rid, buffer, 0, 5, (err: unknown, nwritten: number) => {
|
|
if (err) return reject(err);
|
|
resolve(nwritten);
|
|
});
|
|
});
|
|
|
|
const data = await Deno.readFile(tempFile);
|
|
await Deno.remove(tempFile);
|
|
|
|
assertEquals(bytesWrite, 5);
|
|
assertEquals(decoder.decode(data), "hello");
|
|
},
|
|
});
|
|
|
|
Deno.test({
|
|
name: "Data is written synchronously to the file with the correct length",
|
|
fn() {
|
|
const tempFile: string = Deno.makeTempFileSync();
|
|
using file = Deno.openSync(tempFile, {
|
|
create: true,
|
|
write: true,
|
|
read: true,
|
|
});
|
|
const buffer = Buffer.from("hello world");
|
|
const bytesWrite = writeSync(file.rid, buffer, 0, 5);
|
|
|
|
const data = Deno.readFileSync(tempFile);
|
|
Deno.removeSync(tempFile);
|
|
|
|
assertEquals(bytesWrite, 5);
|
|
assertEquals(decoder.decode(data), "hello");
|
|
},
|
|
});
|