mirror of
https://github.com/denoland/deno
synced 2024-08-25 02:57:22 +00:00
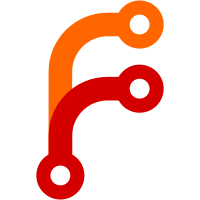
This PR: 1. Replaces `@test_util/std`-prefixed imports with `@std`. 2. Adds `@std/` import map entries to a few `deno.json` files.
101 lines
2.3 KiB
TypeScript
101 lines
2.3 KiB
TypeScript
// Copyright 2018-2024 the Deno authors. All rights reserved. MIT license.
|
|
import { assertEquals, assertThrows, fail } from "@std/assert/mod.ts";
|
|
import { utimes, utimesSync } from "node:fs";
|
|
|
|
const randomDate = new Date(Date.now() + 1000);
|
|
|
|
Deno.test({
|
|
name:
|
|
"ASYNC: change the file system timestamps of the object referenced by path",
|
|
async fn() {
|
|
const file: string = Deno.makeTempFileSync();
|
|
|
|
await new Promise<void>((resolve, reject) => {
|
|
utimes(file, randomDate, randomDate, (err: Error | null) => {
|
|
if (err !== null) reject();
|
|
else resolve();
|
|
});
|
|
})
|
|
.then(
|
|
() => {
|
|
const fileInfo: Deno.FileInfo = Deno.lstatSync(file);
|
|
assertEquals(fileInfo.mtime, randomDate);
|
|
assertEquals(fileInfo.mtime, randomDate);
|
|
},
|
|
() => {
|
|
fail("No error expected");
|
|
},
|
|
)
|
|
.finally(() => Deno.removeSync(file));
|
|
},
|
|
});
|
|
|
|
Deno.test({
|
|
name: "ASYNC: should throw error if atime is infinity",
|
|
fn() {
|
|
assertThrows(
|
|
() => {
|
|
utimes("some/path", Infinity, 0, (_err: Error | null) => {});
|
|
},
|
|
Error,
|
|
"invalid atime, must not be infinity or NaN",
|
|
);
|
|
},
|
|
});
|
|
|
|
Deno.test({
|
|
name: "ASYNC: should throw error if atime is NaN",
|
|
fn() {
|
|
assertThrows(
|
|
() => {
|
|
utimes("some/path", "some string", 0, (_err: Error | null) => {});
|
|
},
|
|
Error,
|
|
"invalid atime, must not be infinity or NaN",
|
|
);
|
|
},
|
|
});
|
|
|
|
Deno.test({
|
|
name:
|
|
"SYNC: change the file system timestamps of the object referenced by path",
|
|
fn() {
|
|
const file: string = Deno.makeTempFileSync();
|
|
try {
|
|
utimesSync(file, randomDate, randomDate);
|
|
|
|
const fileInfo: Deno.FileInfo = Deno.lstatSync(file);
|
|
|
|
assertEquals(fileInfo.mtime, randomDate);
|
|
} finally {
|
|
Deno.removeSync(file);
|
|
}
|
|
},
|
|
});
|
|
|
|
Deno.test({
|
|
name: "SYNC: should throw error if atime is NaN",
|
|
fn() {
|
|
assertThrows(
|
|
() => {
|
|
utimesSync("some/path", "some string", 0);
|
|
},
|
|
Error,
|
|
"invalid atime, must not be infinity or NaN",
|
|
);
|
|
},
|
|
});
|
|
|
|
Deno.test({
|
|
name: "SYNC: should throw error if atime is Infinity",
|
|
fn() {
|
|
assertThrows(
|
|
() => {
|
|
utimesSync("some/path", Infinity, 0);
|
|
},
|
|
Error,
|
|
"invalid atime, must not be infinity or NaN",
|
|
);
|
|
},
|
|
});
|