mirror of
https://github.com/denoland/deno
synced 2024-08-25 02:57:22 +00:00
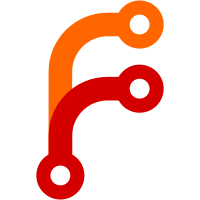
This PR: 1. Replaces `@test_util/std`-prefixed imports with `@std`. 2. Adds `@std/` import map entries to a few `deno.json` files.
93 lines
2.9 KiB
TypeScript
93 lines
2.9 KiB
TypeScript
// Copyright 2018-2024 the Deno authors. All rights reserved. MIT license.
|
|
import { assertEquals, assertNotEquals, fail } from "@std/assert/mod.ts";
|
|
import { assertCallbackErrorUncaught } from "../_test_utils.ts";
|
|
import { readdir, readdirSync } from "node:fs";
|
|
import { join } from "@std/path/mod.ts";
|
|
|
|
Deno.test({
|
|
name: "ASYNC: reading empty directory",
|
|
async fn() {
|
|
const dir = Deno.makeTempDirSync();
|
|
await new Promise<string[]>((resolve, reject) => {
|
|
readdir(dir, (err, files) => {
|
|
if (err) reject(err);
|
|
resolve(files);
|
|
});
|
|
})
|
|
.then((files) => assertEquals(files, []), () => fail())
|
|
.finally(() => Deno.removeSync(dir));
|
|
},
|
|
});
|
|
|
|
function assertEqualsArrayAnyOrder<T>(actual: T[], expected: T[]) {
|
|
assertEquals(actual.length, expected.length);
|
|
for (const item of expected) {
|
|
const index = actual.indexOf(item);
|
|
assertNotEquals(index, -1);
|
|
expected = expected.splice(index, 1);
|
|
}
|
|
}
|
|
|
|
Deno.test({
|
|
name: "ASYNC: reading non-empty directory",
|
|
async fn() {
|
|
const dir = Deno.makeTempDirSync();
|
|
Deno.writeTextFileSync(join(dir, "file1.txt"), "hi");
|
|
Deno.writeTextFileSync(join(dir, "file2.txt"), "hi");
|
|
Deno.mkdirSync(join(dir, "some_dir"));
|
|
await new Promise<string[]>((resolve, reject) => {
|
|
readdir(dir, (err, files) => {
|
|
if (err) reject(err);
|
|
resolve(files);
|
|
});
|
|
})
|
|
.then(
|
|
(files) =>
|
|
assertEqualsArrayAnyOrder(
|
|
files,
|
|
["file1.txt", "some_dir", "file2.txt"],
|
|
),
|
|
() => fail(),
|
|
)
|
|
.finally(() => Deno.removeSync(dir, { recursive: true }));
|
|
},
|
|
});
|
|
|
|
Deno.test({
|
|
name: "SYNC: reading empty the directory",
|
|
fn() {
|
|
const dir = Deno.makeTempDirSync();
|
|
assertEquals(readdirSync(dir), []);
|
|
},
|
|
});
|
|
|
|
Deno.test({
|
|
name: "SYNC: reading non-empty directory",
|
|
fn() {
|
|
const dir = Deno.makeTempDirSync();
|
|
Deno.writeTextFileSync(join(dir, "file1.txt"), "hi");
|
|
Deno.writeTextFileSync(join(dir, "file2.txt"), "hi");
|
|
Deno.mkdirSync(join(dir, "some_dir"));
|
|
assertEqualsArrayAnyOrder(
|
|
readdirSync(dir),
|
|
["file1.txt", "some_dir", "file2.txt"],
|
|
);
|
|
},
|
|
});
|
|
|
|
Deno.test("[std/node/fs] readdir callback isn't called twice if error is thrown", async () => {
|
|
// The correct behaviour is not to catch any errors thrown,
|
|
// but that means there'll be an uncaught error and the test will fail.
|
|
// So the only way to test this is to spawn a subprocess, and succeed if it has a non-zero exit code.
|
|
// (assertRejects won't work because there's no way to catch the error.)
|
|
const tempDir = await Deno.makeTempDir();
|
|
const importUrl = new URL("node:fs", import.meta.url);
|
|
await assertCallbackErrorUncaught({
|
|
prelude: `import { readdir } from ${JSON.stringify(importUrl)}`,
|
|
invocation: `readdir(${JSON.stringify(tempDir)}, `,
|
|
async cleanup() {
|
|
await Deno.remove(tempDir);
|
|
},
|
|
});
|
|
});
|