mirror of
https://github.com/denoland/deno
synced 2024-10-14 03:52:29 +00:00
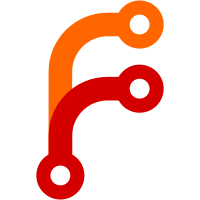
This relates directly to [an
issue](https://github.com/denoland/deno_std/issues/620) that I initially
raised in `deno_std` awhile back, and was reminded about it today when
the `oak` project popped up on my github recommended repos.
As of now Deno's http servers are vulnerable to the same underlying
issue of go CVE-2019-16276 due to the fact that it's based off of ported
go code from their old standard library. [Here's the commit that fixed
the
CVE.](6e6f4aaf70
)
Long story short, some off the shelf proxies and caching servers allow
for passing unaltered malformed headers to backends that they're
fronting. When they pass invalid headers that they don't understand this
can cause issues with HTTP request smuggling. I believe that to this
date, this is the default behavior of AWS ALBs--meaning any server that
strips whitespace from the tail end of header field names and then
interprets the header, when placed behind an ALB, is susceptible to
request smuggling.
The current behavior is actually specifically called out in [RFC
7230](https://tools.ietf.org/html/rfc7230#section-3.2.4) as something
that MUST result in a rejected message, but the change corresponding to
this PR, is more lenient and what both go and nginx currently do, and is
better than the current behavior.
182 lines
4.9 KiB
TypeScript
182 lines
4.9 KiB
TypeScript
// Based on https://github.com/golang/go/blob/master/src/net/textproto/reader_test.go
|
|
// Copyright 2009 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
import { BufReader } from "../io/bufio.ts";
|
|
import { TextProtoReader } from "./mod.ts";
|
|
import { stringsReader } from "../io/util.ts";
|
|
import {
|
|
assert,
|
|
assertEquals,
|
|
assertThrows,
|
|
assertNotEOF,
|
|
} from "../testing/asserts.ts";
|
|
const { test } = Deno;
|
|
|
|
function reader(s: string): TextProtoReader {
|
|
return new TextProtoReader(new BufReader(stringsReader(s)));
|
|
}
|
|
|
|
test({
|
|
ignore: true,
|
|
name: "[textproto] Reader : DotBytes",
|
|
fn(): Promise<void> {
|
|
const _input =
|
|
"dotlines\r\n.foo\r\n..bar\n...baz\nquux\r\n\r\n.\r\nanot.her\r\n";
|
|
return Promise.resolve();
|
|
},
|
|
});
|
|
|
|
test("[textproto] ReadEmpty", async () => {
|
|
const r = reader("");
|
|
const m = await r.readMIMEHeader();
|
|
assertEquals(m, Deno.EOF);
|
|
});
|
|
|
|
test("[textproto] Reader", async () => {
|
|
const r = reader("line1\nline2\n");
|
|
let s = await r.readLine();
|
|
assertEquals(s, "line1");
|
|
|
|
s = await r.readLine();
|
|
assertEquals(s, "line2");
|
|
|
|
s = await r.readLine();
|
|
assert(s === Deno.EOF);
|
|
});
|
|
|
|
test({
|
|
name: "[textproto] Reader : MIME Header",
|
|
async fn(): Promise<void> {
|
|
const input =
|
|
"my-key: Value 1 \r\nLong-key: Even Longer Value\r\nmy-Key: " +
|
|
"Value 2\r\n\n";
|
|
const r = reader(input);
|
|
const m = assertNotEOF(await r.readMIMEHeader());
|
|
assertEquals(m.get("My-Key"), "Value 1, Value 2");
|
|
assertEquals(m.get("Long-key"), "Even Longer Value");
|
|
},
|
|
});
|
|
|
|
test({
|
|
name: "[textproto] Reader : MIME Header Single",
|
|
async fn(): Promise<void> {
|
|
const input = "Foo: bar\n\n";
|
|
const r = reader(input);
|
|
const m = assertNotEOF(await r.readMIMEHeader());
|
|
assertEquals(m.get("Foo"), "bar");
|
|
},
|
|
});
|
|
|
|
test({
|
|
name: "[textproto] Reader : MIME Header No Key",
|
|
async fn(): Promise<void> {
|
|
const input = ": bar\ntest-1: 1\n\n";
|
|
const r = reader(input);
|
|
const m = assertNotEOF(await r.readMIMEHeader());
|
|
assertEquals(m.get("Test-1"), "1");
|
|
},
|
|
});
|
|
|
|
test({
|
|
name: "[textproto] Reader : Large MIME Header",
|
|
async fn(): Promise<void> {
|
|
const data: string[] = [];
|
|
// Go test is 16*1024. But seems it can't handle more
|
|
for (let i = 0; i < 1024; i++) {
|
|
data.push("x");
|
|
}
|
|
const sdata = data.join("");
|
|
const r = reader(`Cookie: ${sdata}\r\n\r\n`);
|
|
const m = assertNotEOF(await r.readMIMEHeader());
|
|
assertEquals(m.get("Cookie"), sdata);
|
|
},
|
|
});
|
|
|
|
// Test that we don't read MIME headers seen in the wild,
|
|
// with spaces before colons, and spaces in keys.
|
|
test({
|
|
name: "[textproto] Reader : MIME Header Non compliant",
|
|
async fn(): Promise<void> {
|
|
const input =
|
|
"Foo: bar\r\n" +
|
|
"Content-Language: en\r\n" +
|
|
"SID : 0\r\n" +
|
|
"Audio Mode : None\r\n" +
|
|
"Privilege : 127\r\n\r\n";
|
|
const r = reader(input);
|
|
const m = assertNotEOF(await r.readMIMEHeader());
|
|
assertEquals(m.get("Foo"), "bar");
|
|
assertEquals(m.get("Content-Language"), "en");
|
|
// Make sure we drop headers with trailing whitespace
|
|
assertEquals(m.get("SID"), null);
|
|
assertEquals(m.get("Privilege"), null);
|
|
// Not legal http header
|
|
assertThrows((): void => {
|
|
assertEquals(m.get("Audio Mode"), "None");
|
|
});
|
|
},
|
|
});
|
|
|
|
test({
|
|
name: "[textproto] Reader : MIME Header Malformed",
|
|
async fn(): Promise<void> {
|
|
const input = [
|
|
"No colon first line\r\nFoo: foo\r\n\r\n",
|
|
" No colon first line with leading space\r\nFoo: foo\r\n\r\n",
|
|
"\tNo colon first line with leading tab\r\nFoo: foo\r\n\r\n",
|
|
" First: line with leading space\r\nFoo: foo\r\n\r\n",
|
|
"\tFirst: line with leading tab\r\nFoo: foo\r\n\r\n",
|
|
"Foo: foo\r\nNo colon second line\r\n\r\n",
|
|
];
|
|
const r = reader(input.join(""));
|
|
|
|
let err;
|
|
try {
|
|
await r.readMIMEHeader();
|
|
} catch (e) {
|
|
err = e;
|
|
}
|
|
assert(err instanceof Deno.errors.InvalidData);
|
|
},
|
|
});
|
|
|
|
test({
|
|
name: "[textproto] Reader : MIME Header Trim Continued",
|
|
async fn(): Promise<void> {
|
|
const input =
|
|
"" + // for code formatting purpose.
|
|
"a:\n" +
|
|
" 0 \r\n" +
|
|
"b:1 \t\r\n" +
|
|
"c: 2\r\n" +
|
|
" 3\t\n" +
|
|
" \t 4 \r\n\n";
|
|
const r = reader(input);
|
|
let err;
|
|
try {
|
|
await r.readMIMEHeader();
|
|
} catch (e) {
|
|
err = e;
|
|
}
|
|
assert(err instanceof Deno.errors.InvalidData);
|
|
},
|
|
});
|
|
|
|
test({
|
|
name: "[textproto] #409 issue : multipart form boundary",
|
|
async fn(): Promise<void> {
|
|
const input = [
|
|
"Accept: */*\r\n",
|
|
'Content-Disposition: form-data; name="test"\r\n',
|
|
" \r\n",
|
|
"------WebKitFormBoundaryimeZ2Le9LjohiUiG--\r\n\n",
|
|
];
|
|
const r = reader(input.join(""));
|
|
const m = assertNotEOF(await r.readMIMEHeader());
|
|
assertEquals(m.get("Accept"), "*/*");
|
|
assertEquals(m.get("Content-Disposition"), 'form-data; name="test"');
|
|
},
|
|
});
|