mirror of
https://github.com/denoland/deno
synced 2024-10-13 11:32:29 +00:00
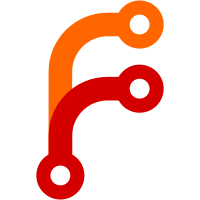
* Changed tools/lint.py to lint the entire js and tests directorys and sub directories, currently it was pointing at tsconfig and would only lint files that were part of js/main.ts or node_modules/typescript/lib/lib.esnext.d.ts and their dependencies * Broke the typescript linting out into separate steps for the main typescript programing and tests. * Fixed linting issues in ts tests.
61 lines
1.4 KiB
TypeScript
61 lines
1.4 KiB
TypeScript
// Copyright 2018 the Deno authors. All rights reserved. MIT license.
|
|
import { test, testPerm, assert, assertEqual } from "./test_util.ts";
|
|
import * as deno from "deno";
|
|
import { FileInfo } from "deno";
|
|
|
|
function assertSameContent(files: FileInfo[]) {
|
|
let counter = 0;
|
|
|
|
for (const file of files) {
|
|
if (file.name === "subdir") {
|
|
assert(file.isDirectory());
|
|
counter++;
|
|
}
|
|
|
|
if (file.name === "002_hello.ts") {
|
|
assertEqual(file.path, `tests/${file.name}`);
|
|
counter++;
|
|
}
|
|
}
|
|
|
|
assertEqual(counter, 2);
|
|
}
|
|
|
|
testPerm({ write: true }, function readDirSyncSuccess() {
|
|
const files = deno.readDirSync("tests/");
|
|
assertSameContent(files);
|
|
});
|
|
|
|
test(function readDirSyncNotDir() {
|
|
let caughtError = false;
|
|
let src;
|
|
|
|
try {
|
|
src = deno.readDirSync("package.json");
|
|
} catch (err) {
|
|
caughtError = true;
|
|
assertEqual(err.kind, deno.ErrorKind.Other);
|
|
}
|
|
assert(caughtError);
|
|
assertEqual(src, undefined);
|
|
});
|
|
|
|
test(function readDirSyncNotFound() {
|
|
let caughtError = false;
|
|
let src;
|
|
|
|
try {
|
|
src = deno.readDirSync("bad_dir_name");
|
|
} catch (err) {
|
|
caughtError = true;
|
|
assertEqual(err.kind, deno.ErrorKind.NotFound);
|
|
}
|
|
assert(caughtError);
|
|
assertEqual(src, undefined);
|
|
});
|
|
|
|
testPerm({ write: true }, async function readDirSuccess() {
|
|
const files = await deno.readDir("tests/");
|
|
assertSameContent(files);
|
|
});
|