mirror of
https://github.com/denoland/deno
synced 2024-08-25 02:57:22 +00:00
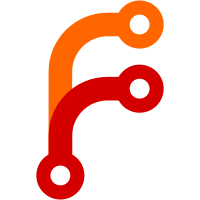
This is a follow-on to the earlier work in reducing string copies, mainly focused on ensuring that ASCII strings are easy to provide to the JS runtime. While we are replacing a 16-byte reference in a number of places with a 24-byte structure (measured via `std::mem::size_of`), the reduction in copies wins out over the additional size of the arguments passed into functions. Benchmarking shows approximately the same if not slightly less wallclock time/instructions retired, but I believe this continues to open up further refactoring opportunities.
37 lines
840 B
Rust
37 lines
840 B
Rust
// Copyright 2018-2023 the Deno authors. All rights reserved. MIT license.
|
|
|
|
use deno_core::Snapshot;
|
|
use log::debug;
|
|
|
|
static CLI_SNAPSHOT: &[u8] =
|
|
include_bytes!(concat!(env!("OUT_DIR"), "/CLI_SNAPSHOT.bin"));
|
|
|
|
pub fn deno_isolate_init() -> Snapshot {
|
|
debug!("Deno isolate init with snapshots.");
|
|
Snapshot::Static(CLI_SNAPSHOT)
|
|
}
|
|
|
|
#[cfg(test)]
|
|
mod tests {
|
|
use super::*;
|
|
|
|
#[test]
|
|
fn runtime_snapshot() {
|
|
let mut js_runtime = deno_core::JsRuntime::new(deno_core::RuntimeOptions {
|
|
startup_snapshot: Some(deno_isolate_init()),
|
|
..Default::default()
|
|
});
|
|
js_runtime
|
|
.execute_script_static(
|
|
"<anon>",
|
|
r#"
|
|
if (!(bootstrap.mainRuntime && bootstrap.workerRuntime)) {
|
|
throw Error("bad");
|
|
}
|
|
console.log("we have console.log!!!");
|
|
"#,
|
|
)
|
|
.unwrap();
|
|
}
|
|
}
|