mirror of
https://github.com/denoland/deno
synced 2024-09-13 21:31:18 +00:00
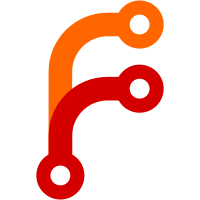
This looks like a massive PR, but it's only a move from cli/tests -> tests, and updates of relative paths for files. This is the first step towards aggregate all of the integration test files under tests/, which will lead to a set of integration tests that can run without the CLI binary being built. While we could leave these tests under `cli`, it would require us to keep a more complex directory structure for the various test runners. In addition, we have a lot of complexity to ignore various test files in the `cli` project itself (cargo publish exclusion rules, autotests = false, etc). And finally, the `tests/` folder will eventually house the `test_ffi`, `test_napi` and other testing code, reducing the size of the root repo directory. For easier review, the extremely large and noisy "move" is in the first commit (with no changes -- just a move), while the remainder of the changes to actual files is in the second commit.
144 lines
5.2 KiB
TypeScript
144 lines
5.2 KiB
TypeScript
// Copyright 2018-2024 the Deno authors. All rights reserved. MIT license.
|
|
import { assertEquals, assertStringIncludes } from "./test_util.ts";
|
|
|
|
Deno.test(function eventInitializedWithType() {
|
|
const type = "click";
|
|
const event = new Event(type);
|
|
|
|
assertEquals(event.isTrusted, false);
|
|
assertEquals(event.target, null);
|
|
assertEquals(event.currentTarget, null);
|
|
assertEquals(event.type, "click");
|
|
assertEquals(event.bubbles, false);
|
|
assertEquals(event.cancelable, false);
|
|
});
|
|
|
|
Deno.test(function eventInitializedWithTypeAndDict() {
|
|
const init = "submit";
|
|
const eventInit = { bubbles: true, cancelable: true } as EventInit;
|
|
const event = new Event(init, eventInit);
|
|
|
|
assertEquals(event.isTrusted, false);
|
|
assertEquals(event.target, null);
|
|
assertEquals(event.currentTarget, null);
|
|
assertEquals(event.type, "submit");
|
|
assertEquals(event.bubbles, true);
|
|
assertEquals(event.cancelable, true);
|
|
});
|
|
|
|
Deno.test(function eventComposedPathSuccess() {
|
|
const type = "click";
|
|
const event = new Event(type);
|
|
const composedPath = event.composedPath();
|
|
|
|
assertEquals(composedPath, []);
|
|
});
|
|
|
|
Deno.test(function eventStopPropagationSuccess() {
|
|
const type = "click";
|
|
const event = new Event(type);
|
|
|
|
assertEquals(event.cancelBubble, false);
|
|
event.stopPropagation();
|
|
assertEquals(event.cancelBubble, true);
|
|
});
|
|
|
|
Deno.test(function eventStopImmediatePropagationSuccess() {
|
|
const type = "click";
|
|
const event = new Event(type);
|
|
|
|
assertEquals(event.cancelBubble, false);
|
|
event.stopImmediatePropagation();
|
|
assertEquals(event.cancelBubble, true);
|
|
});
|
|
|
|
Deno.test(function eventPreventDefaultSuccess() {
|
|
const type = "click";
|
|
const event = new Event(type);
|
|
|
|
assertEquals(event.defaultPrevented, false);
|
|
event.preventDefault();
|
|
assertEquals(event.defaultPrevented, false);
|
|
|
|
const eventInit = { bubbles: true, cancelable: true } as EventInit;
|
|
const cancelableEvent = new Event(type, eventInit);
|
|
assertEquals(cancelableEvent.defaultPrevented, false);
|
|
cancelableEvent.preventDefault();
|
|
assertEquals(cancelableEvent.defaultPrevented, true);
|
|
});
|
|
|
|
Deno.test(function eventInitializedWithNonStringType() {
|
|
// deno-lint-ignore no-explicit-any
|
|
const type: any = undefined;
|
|
const event = new Event(type);
|
|
|
|
assertEquals(event.isTrusted, false);
|
|
assertEquals(event.target, null);
|
|
assertEquals(event.currentTarget, null);
|
|
assertEquals(event.type, "undefined");
|
|
assertEquals(event.bubbles, false);
|
|
assertEquals(event.cancelable, false);
|
|
});
|
|
|
|
Deno.test(function eventInspectOutput() {
|
|
// deno-lint-ignore no-explicit-any
|
|
const cases: Array<[any, (event: any) => string]> = [
|
|
[
|
|
new Event("test"),
|
|
(event: Event) =>
|
|
`Event {\n bubbles: false,\n cancelable: false,\n composed: false,\n currentTarget: null,\n defaultPrevented: false,\n eventPhase: 0,\n srcElement: null,\n target: null,\n returnValue: true,\n timeStamp: ${event.timeStamp},\n type: "test"\n}`,
|
|
],
|
|
[
|
|
new ErrorEvent("error"),
|
|
(event: Event) =>
|
|
`ErrorEvent {\n bubbles: false,\n cancelable: false,\n composed: false,\n currentTarget: null,\n defaultPrevented: false,\n eventPhase: 0,\n srcElement: null,\n target: null,\n returnValue: true,\n timeStamp: ${event.timeStamp},\n type: "error",\n message: "",\n filename: "",\n lineno: 0,\n colno: 0,\n error: undefined\n}`,
|
|
],
|
|
[
|
|
new CloseEvent("close"),
|
|
(event: Event) =>
|
|
`CloseEvent {\n bubbles: false,\n cancelable: false,\n composed: false,\n currentTarget: null,\n defaultPrevented: false,\n eventPhase: 0,\n srcElement: null,\n target: null,\n returnValue: true,\n timeStamp: ${event.timeStamp},\n type: "close",\n wasClean: false,\n code: 0,\n reason: ""\n}`,
|
|
],
|
|
[
|
|
new CustomEvent("custom"),
|
|
(event: Event) =>
|
|
`CustomEvent {\n bubbles: false,\n cancelable: false,\n composed: false,\n currentTarget: null,\n defaultPrevented: false,\n eventPhase: 0,\n srcElement: null,\n target: null,\n returnValue: true,\n timeStamp: ${event.timeStamp},\n type: "custom",\n detail: undefined\n}`,
|
|
],
|
|
[
|
|
new ProgressEvent("progress"),
|
|
(event: Event) =>
|
|
`ProgressEvent {\n bubbles: false,\n cancelable: false,\n composed: false,\n currentTarget: null,\n defaultPrevented: false,\n eventPhase: 0,\n srcElement: null,\n target: null,\n returnValue: true,\n timeStamp: ${event.timeStamp},\n type: "progress",\n lengthComputable: false,\n loaded: 0,\n total: 0\n}`,
|
|
],
|
|
];
|
|
|
|
for (const [event, outputProvider] of cases) {
|
|
assertEquals(Deno.inspect(event), outputProvider(event));
|
|
}
|
|
});
|
|
|
|
Deno.test(function inspectEvent() {
|
|
// has a customInspect implementation that previously would throw on a getter
|
|
assertEquals(
|
|
Deno.inspect(Event.prototype),
|
|
`Event {
|
|
bubbles: [Getter],
|
|
cancelable: [Getter],
|
|
composed: [Getter],
|
|
currentTarget: [Getter],
|
|
defaultPrevented: [Getter],
|
|
eventPhase: [Getter],
|
|
srcElement: [Getter/Setter],
|
|
target: [Getter],
|
|
returnValue: [Getter/Setter],
|
|
timeStamp: [Getter],
|
|
type: [Getter]
|
|
}`,
|
|
);
|
|
|
|
// ensure this still works
|
|
assertStringIncludes(
|
|
Deno.inspect(new Event("test")),
|
|
// check a substring because one property is a timestamp
|
|
`Event {\n bubbles: false,\n cancelable: false,`,
|
|
);
|
|
});
|