mirror of
https://github.com/denoland/deno
synced 2024-10-13 11:32:29 +00:00
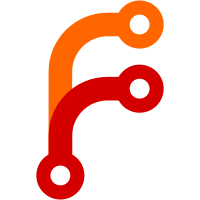
This commit adds a "--no-check" option to following subcommands: - "deno cache" - "deno info" - "deno run" - "deno test" The "--no-check" options allows to skip type checking step and instead directly transpiles TS sources to JS sources. This solution uses `ts.transpileModule()` API and is just an interim solution before implementing it fully in Rust.
35 lines
692 B
TypeScript
35 lines
692 B
TypeScript
// Copyright 2018-2020 the Deno authors. All rights reserved. MIT license.
|
|
|
|
import { Hash } from "./_wasm/hash.ts";
|
|
import type { Hasher } from "./hasher.ts";
|
|
|
|
export type { Hasher } from "./hasher.ts";
|
|
export type SupportedAlgorithm =
|
|
| "md2"
|
|
| "md4"
|
|
| "md5"
|
|
| "ripemd160"
|
|
| "ripemd320"
|
|
| "sha1"
|
|
| "sha224"
|
|
| "sha256"
|
|
| "sha384"
|
|
| "sha512"
|
|
| "sha3-224"
|
|
| "sha3-256"
|
|
| "sha3-384"
|
|
| "sha3-512"
|
|
| "keccak224"
|
|
| "keccak256"
|
|
| "keccak384"
|
|
| "keccak512";
|
|
|
|
/**
|
|
* Creates a new `Hash` instance.
|
|
*
|
|
* @param algorithm name of hash algorithm to use
|
|
*/
|
|
export function createHash(algorithm: SupportedAlgorithm): Hasher {
|
|
return new Hash(algorithm as string);
|
|
}
|