mirror of
https://github.com/denoland/deno
synced 2024-08-27 20:09:17 +00:00
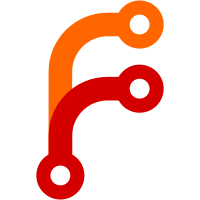
Resolves #1705 This PR adds the Deno APIs as a global namespace named `Deno`. For backwards compatibility, the ability to `import * from "deno"` is preserved. I have tried to convert every test and internal code the references the module to use the namespace instead, but because I didn't break compatibility I am not sure. On the REPL, `deno` no longer exists, replaced only with `Deno` to align with the regular runtime. The runtime type library includes both the namespace and module. This means it duplicates the whole type information. When we remove the functionality from the runtime, it will be a one line change to the library generator to remove the module definition from the type library. I marked a `TODO` in a couple places where to remove the `"deno"` module, but there are additional places I know I didn't mark.
35 lines
873 B
TypeScript
35 lines
873 B
TypeScript
// Used for benchmarking Deno's networking. See tools/http_benchmark.py
|
|
// TODO Replace this with a real HTTP server once
|
|
// https://github.com/denoland/deno/issues/726 is completed.
|
|
// Note: this is a keep-alive server.
|
|
const addr = Deno.args[1] || "127.0.0.1:4500";
|
|
const listener = Deno.listen("tcp", addr);
|
|
const response = new TextEncoder().encode(
|
|
"HTTP/1.1 200 OK\r\nContent-Length: 12\r\n\r\nHello World\n"
|
|
);
|
|
|
|
async function handle(conn: Deno.Conn): Promise<void> {
|
|
const buffer = new Uint8Array(1024);
|
|
try {
|
|
while (true) {
|
|
const r = await conn.read(buffer);
|
|
if (r.eof) {
|
|
break;
|
|
}
|
|
await conn.write(response);
|
|
}
|
|
} finally {
|
|
conn.close();
|
|
}
|
|
}
|
|
|
|
async function main(): Promise<void> {
|
|
console.log("Listening on", addr);
|
|
while (true) {
|
|
const conn = await listener.accept();
|
|
handle(conn);
|
|
}
|
|
}
|
|
|
|
main();
|