mirror of
https://github.com/denoland/deno
synced 2024-09-13 05:11:43 +00:00
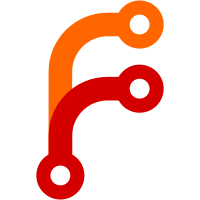
This looks like a massive PR, but it's only a move from cli/tests -> tests, and updates of relative paths for files. This is the first step towards aggregate all of the integration test files under tests/, which will lead to a set of integration tests that can run without the CLI binary being built. While we could leave these tests under `cli`, it would require us to keep a more complex directory structure for the various test runners. In addition, we have a lot of complexity to ignore various test files in the `cli` project itself (cargo publish exclusion rules, autotests = false, etc). And finally, the `tests/` folder will eventually house the `test_ffi`, `test_napi` and other testing code, reducing the size of the root repo directory. For easier review, the extremely large and noisy "move" is in the first commit (with no changes -- just a move), while the remainder of the changes to actual files is in the second commit.
155 lines
3.8 KiB
TypeScript
155 lines
3.8 KiB
TypeScript
// Copyright 2018-2024 the Deno authors. All rights reserved. MIT license.
|
|
import { assertEquals, assertRejects, assertThrows } from "./test_util.ts";
|
|
|
|
Deno.test(function testWrongOverloads() {
|
|
assertThrows(
|
|
() => {
|
|
// @ts-ignore Testing invalid overloads
|
|
Deno.test("some name", { fn: () => {} }, () => {});
|
|
},
|
|
TypeError,
|
|
"Unexpected 'fn' field in options, test function is already provided as the third argument.",
|
|
);
|
|
assertThrows(
|
|
() => {
|
|
// @ts-ignore Testing invalid overloads
|
|
Deno.test("some name", { name: "some name2" }, () => {});
|
|
},
|
|
TypeError,
|
|
"Unexpected 'name' field in options, test name is already provided as the first argument.",
|
|
);
|
|
assertThrows(
|
|
() => {
|
|
// @ts-ignore Testing invalid overloads
|
|
Deno.test(() => {});
|
|
},
|
|
TypeError,
|
|
"The test function must have a name",
|
|
);
|
|
assertThrows(
|
|
() => {
|
|
// @ts-ignore Testing invalid overloads
|
|
Deno.test(function foo() {}, {});
|
|
},
|
|
TypeError,
|
|
"Unexpected second argument to Deno.test()",
|
|
);
|
|
assertThrows(
|
|
() => {
|
|
// @ts-ignore Testing invalid overloads
|
|
Deno.test({ fn: () => {} }, function foo() {});
|
|
},
|
|
TypeError,
|
|
"Unexpected 'fn' field in options, test function is already provided as the second argument.",
|
|
);
|
|
assertThrows(
|
|
() => {
|
|
// @ts-ignore Testing invalid overloads
|
|
Deno.test({});
|
|
},
|
|
TypeError,
|
|
"Expected 'fn' field in the first argument to be a test function.",
|
|
);
|
|
assertThrows(
|
|
() => {
|
|
// @ts-ignore Testing invalid overloads
|
|
Deno.test({ fn: "boo!" });
|
|
},
|
|
TypeError,
|
|
"Expected 'fn' field in the first argument to be a test function.",
|
|
);
|
|
});
|
|
|
|
Deno.test(function nameOfTestCaseCantBeEmpty() {
|
|
assertThrows(
|
|
() => {
|
|
Deno.test("", () => {});
|
|
},
|
|
TypeError,
|
|
"The test name can't be empty",
|
|
);
|
|
assertThrows(
|
|
() => {
|
|
Deno.test({
|
|
name: "",
|
|
fn: () => {},
|
|
});
|
|
},
|
|
TypeError,
|
|
"The test name can't be empty",
|
|
);
|
|
});
|
|
|
|
Deno.test(async function invalidStepArguments(t) {
|
|
await assertRejects(
|
|
async () => {
|
|
// deno-lint-ignore no-explicit-any
|
|
await (t as any).step("test");
|
|
},
|
|
TypeError,
|
|
"Expected function for second argument.",
|
|
);
|
|
|
|
await assertRejects(
|
|
async () => {
|
|
// deno-lint-ignore no-explicit-any
|
|
await (t as any).step("test", "not a function");
|
|
},
|
|
TypeError,
|
|
"Expected function for second argument.",
|
|
);
|
|
|
|
await assertRejects(
|
|
async () => {
|
|
// deno-lint-ignore no-explicit-any
|
|
await (t as any).step();
|
|
},
|
|
TypeError,
|
|
"Expected a test definition or name and function.",
|
|
);
|
|
|
|
await assertRejects(
|
|
async () => {
|
|
// deno-lint-ignore no-explicit-any
|
|
await (t as any).step(() => {});
|
|
},
|
|
TypeError,
|
|
"The step function must have a name.",
|
|
);
|
|
});
|
|
|
|
Deno.test(async function nameOnTextContext(t1) {
|
|
await assertEquals(t1.name, "nameOnTextContext");
|
|
await t1.step("step", async (t2) => {
|
|
await assertEquals(t2.name, "step");
|
|
await t2.step("nested step", async (t3) => {
|
|
await assertEquals(t3.name, "nested step");
|
|
});
|
|
});
|
|
});
|
|
|
|
Deno.test(async function originOnTextContext(t1) {
|
|
await assertEquals(t1.origin, Deno.mainModule);
|
|
await t1.step("step", async (t2) => {
|
|
await assertEquals(t2.origin, Deno.mainModule);
|
|
await t2.step("nested step", async (t3) => {
|
|
await assertEquals(t3.origin, Deno.mainModule);
|
|
});
|
|
});
|
|
});
|
|
|
|
Deno.test(async function parentOnTextContext(t1) {
|
|
await assertEquals(t1.parent, undefined);
|
|
await t1.step("step", async (t2) => {
|
|
await assertEquals(t1, t2.parent);
|
|
await t2.step("nested step", async (t3) => {
|
|
await assertEquals(t2, t3.parent);
|
|
});
|
|
});
|
|
});
|
|
|
|
Deno.test("explicit undefined for boolean options", {
|
|
ignore: undefined,
|
|
only: undefined,
|
|
}, () => {});
|