mirror of
https://github.com/rust-lang/cargo
synced 2024-10-01 13:33:38 +00:00
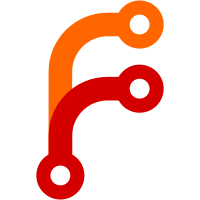
This test has been flaky on the bots for quite some time now, and the cause has now been discovered. The root cause of the failure is that the execve for the `cargo --list` command was failing with ETXTBUSY. In querying the manpage, this means: Executable was open for writing by one or more processes. This error can be explained by the following trace: 1. Thread A, running the `cargo --list` test, opens the destination executable for writing because it's copying the current executable into a different location. 2. Thread B, some other test, forks the process. The file descriptor of the destination executable of thread A is now duplicated in this process. 3. Thread A closes all files and such, and then goes to fork/exec `cargo --list`. 4. Thread B has not had time to close all its descriptors. so it still has the executable open for writing, causing the `execve` of thread A to fail. This commit just removes these tested portions of the test, only testing that cargo probes PATH.
47 lines
1.8 KiB
Rust
47 lines
1.8 KiB
Rust
use std::io::fs;
|
|
use std::io;
|
|
use std::os;
|
|
use std::str;
|
|
use cargo::util::process;
|
|
|
|
use support::paths;
|
|
use support::{project, cargo_dir, mkdir_recursive, ProjectBuilder, ResultTest};
|
|
|
|
fn setup() {
|
|
}
|
|
|
|
/// Add an empty file with executable flags (and platform-dependent suffix).
|
|
/// TODO: move this to `ProjectBuilder` if other cases using this emerge.
|
|
fn fake_executable(proj: ProjectBuilder, dir: &Path, name: &str) -> ProjectBuilder {
|
|
let path = proj.root().join(dir).join(format!("{}{}", name, os::consts::EXE_SUFFIX));
|
|
mkdir_recursive(&Path::new(path.dirname())).assert();
|
|
fs::File::create(&path).assert();
|
|
let io::FileStat{perm, ..} = fs::stat(&path).assert();
|
|
fs::chmod(&path, io::OtherExecute | perm).assert();
|
|
proj
|
|
}
|
|
|
|
// We can't entirely obliterate PATH because windows needs it for paths to
|
|
// things like libgcc, but we want to filter out everything which has a `cargo`
|
|
// installation as we don't want it to muck with the --list tests
|
|
fn new_path() -> Vec<Path> {
|
|
let path = os::getenv_as_bytes("PATH").unwrap_or(Vec::new());
|
|
os::split_paths(path).move_iter().filter(|p| {
|
|
!p.join(format!("cargo{}", os::consts::EXE_SUFFIX)).exists()
|
|
}).collect()
|
|
}
|
|
test!(list_commands_looks_at_path {
|
|
let proj = project("list-non-overlapping");
|
|
let proj = fake_executable(proj, &Path::new("path-test"), "cargo-1");
|
|
let pr = process(cargo_dir().join("cargo")).cwd(proj.root())
|
|
.env("HOME", Some(paths::home()));
|
|
|
|
let mut path = new_path();
|
|
path.push(proj.root().join("path-test"));
|
|
let path = os::join_paths(path.as_slice()).unwrap();
|
|
let output = pr.arg("-v").arg("--list").env("PATH", Some(path.as_slice()));
|
|
let output = output.exec_with_output().assert();
|
|
let output = str::from_utf8(output.output.as_slice()).assert();
|
|
assert!(output.contains("\n 1\n"), "missing 1: {}", output);
|
|
})
|