mirror of
https://github.com/rust-lang/cargo
synced 2024-10-13 19:22:33 +00:00
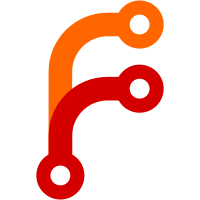
This is an alternative to `-Zminimal-versions` as discussed in #5657. The problem with `-Zminimal-versions` is it requires the root most dependencies to verify it and we then percolate that up the stack. This requires a massive level of cooperation to accomplish and so far there have been mixed results with it to the point that cargo's unstable documentation discourages its use. `-Zdirect-minimal-versions` instead only applies this rule to your direct dependencies, allowing anyone in the stack to immediately adopt it, independent of everyone else. Special notes - Living up to the name and the existing design, this ignores yanked crates. This makes sense for `^1.1` version requirements but might look weird for `^1.1.1` version requirements as it could select `1.1.2`. - This will error if an indirect dependency requires a newer version. Your version requirement will need to capture what you use **and** all of you dependencies. An alternative design would have tried to merge the result of minimum versions for direct dependencies and maximum versions for indirect dependencies. This would have been complex and led to weird corner cases, making it harder to predict. I also suspect the value gained would be relatively low as you can't verify that version requirement in any other way. - The error could be improved to call out that this was from minimal versions but I felt getting this out now and starting to collect feedback was more important.
237 lines
6 KiB
Rust
237 lines
6 KiB
Rust
//! Tests for minimal-version resolution.
|
|
//!
|
|
//! Note: Some tests are located in the resolver-tests package.
|
|
|
|
use cargo_test_support::project;
|
|
use cargo_test_support::registry::Package;
|
|
|
|
#[cargo_test]
|
|
fn simple() {
|
|
Package::new("dep", "1.0.0").publish();
|
|
Package::new("dep", "1.1.0").publish();
|
|
|
|
let p = project()
|
|
.file(
|
|
"Cargo.toml",
|
|
r#"
|
|
[package]
|
|
name = "foo"
|
|
authors = []
|
|
version = "0.0.1"
|
|
|
|
[dependencies]
|
|
dep = "1.0"
|
|
"#,
|
|
)
|
|
.file("src/main.rs", "fn main() {}")
|
|
.build();
|
|
|
|
p.cargo("generate-lockfile -Zdirect-minimal-versions")
|
|
.masquerade_as_nightly_cargo(&["direct-minimal-versions"])
|
|
.run();
|
|
|
|
let lock = p.read_lockfile();
|
|
|
|
assert!(
|
|
lock.contains("1.0.0"),
|
|
"dep minimal version must be present"
|
|
);
|
|
assert!(
|
|
!lock.contains("1.1.0"),
|
|
"dep maximimal version cannot be present"
|
|
);
|
|
}
|
|
|
|
#[cargo_test]
|
|
fn mixed_dependencies() {
|
|
Package::new("dep", "1.0.0").publish();
|
|
Package::new("dep", "1.1.0").publish();
|
|
Package::new("dep", "1.2.0").publish();
|
|
|
|
let p = project()
|
|
.file(
|
|
"Cargo.toml",
|
|
r#"
|
|
[package]
|
|
name = "foo"
|
|
authors = []
|
|
version = "0.0.1"
|
|
|
|
[dependencies]
|
|
dep = "1.0"
|
|
|
|
[dev-dependencies]
|
|
dep = "1.1"
|
|
"#,
|
|
)
|
|
.file("src/main.rs", "fn main() {}")
|
|
.build();
|
|
|
|
p.cargo("generate-lockfile -Zdirect-minimal-versions")
|
|
.masquerade_as_nightly_cargo(&["direct-minimal-versions"])
|
|
.with_status(101)
|
|
.with_stderr(
|
|
r#"[UPDATING] [..]
|
|
[ERROR] failed to select a version for `dep`.
|
|
... required by package `foo v0.0.1 ([CWD])`
|
|
versions that meet the requirements `^1.1` are: 1.1.0
|
|
|
|
all possible versions conflict with previously selected packages.
|
|
|
|
previously selected package `dep v1.0.0`
|
|
... which satisfies dependency `dep = "^1.0"` of package `foo v0.0.1 ([CWD])`
|
|
|
|
failed to select a version for `dep` which could resolve this conflict
|
|
"#,
|
|
)
|
|
.run();
|
|
}
|
|
|
|
#[cargo_test]
|
|
fn yanked() {
|
|
Package::new("dep", "1.0.0").yanked(true).publish();
|
|
Package::new("dep", "1.1.0").publish();
|
|
Package::new("dep", "1.2.0").publish();
|
|
|
|
let p = project()
|
|
.file(
|
|
"Cargo.toml",
|
|
r#"
|
|
[package]
|
|
name = "foo"
|
|
authors = []
|
|
version = "0.0.1"
|
|
|
|
[dependencies]
|
|
dep = "1.0"
|
|
"#,
|
|
)
|
|
.file("src/main.rs", "fn main() {}")
|
|
.build();
|
|
|
|
p.cargo("generate-lockfile -Zdirect-minimal-versions")
|
|
.masquerade_as_nightly_cargo(&["direct-minimal-versions"])
|
|
.run();
|
|
|
|
let lock = p.read_lockfile();
|
|
|
|
assert!(
|
|
lock.contains("1.1.0"),
|
|
"dep minimal version must be present"
|
|
);
|
|
assert!(
|
|
!lock.contains("1.0.0"),
|
|
"yanked minimal version must be skipped"
|
|
);
|
|
assert!(
|
|
!lock.contains("1.2.0"),
|
|
"dep maximimal version cannot be present"
|
|
);
|
|
}
|
|
|
|
#[cargo_test]
|
|
fn indirect() {
|
|
Package::new("indirect", "2.0.0").publish();
|
|
Package::new("indirect", "2.1.0").publish();
|
|
Package::new("indirect", "2.2.0").publish();
|
|
Package::new("direct", "1.0.0")
|
|
.dep("indirect", "2.1")
|
|
.publish();
|
|
Package::new("direct", "1.1.0")
|
|
.dep("indirect", "2.1")
|
|
.publish();
|
|
|
|
let p = project()
|
|
.file(
|
|
"Cargo.toml",
|
|
r#"
|
|
[package]
|
|
name = "foo"
|
|
authors = []
|
|
version = "0.0.1"
|
|
|
|
[dependencies]
|
|
direct = "1.0"
|
|
"#,
|
|
)
|
|
.file("src/main.rs", "fn main() {}")
|
|
.build();
|
|
|
|
p.cargo("generate-lockfile -Zdirect-minimal-versions")
|
|
.masquerade_as_nightly_cargo(&["direct-minimal-versions"])
|
|
.run();
|
|
|
|
let lock = p.read_lockfile();
|
|
|
|
assert!(
|
|
lock.contains("1.0.0"),
|
|
"direct minimal version must be present"
|
|
);
|
|
assert!(
|
|
!lock.contains("1.1.0"),
|
|
"direct maximimal version cannot be present"
|
|
);
|
|
assert!(
|
|
!lock.contains("2.0.0"),
|
|
"indirect minimal version cannot be present"
|
|
);
|
|
assert!(
|
|
!lock.contains("2.1.0"),
|
|
"indirect minimal version cannot be present"
|
|
);
|
|
assert!(
|
|
lock.contains("2.2.0"),
|
|
"indirect maximal version must be present"
|
|
);
|
|
}
|
|
|
|
#[cargo_test]
|
|
fn indirect_conflict() {
|
|
Package::new("indirect", "2.0.0").publish();
|
|
Package::new("indirect", "2.1.0").publish();
|
|
Package::new("indirect", "2.2.0").publish();
|
|
Package::new("direct", "1.0.0")
|
|
.dep("indirect", "2.1")
|
|
.publish();
|
|
Package::new("direct", "1.1.0")
|
|
.dep("indirect", "2.1")
|
|
.publish();
|
|
|
|
let p = project()
|
|
.file(
|
|
"Cargo.toml",
|
|
r#"
|
|
[package]
|
|
name = "foo"
|
|
authors = []
|
|
version = "0.0.1"
|
|
|
|
[dependencies]
|
|
direct = "1.0"
|
|
indirect = "2.0"
|
|
"#,
|
|
)
|
|
.file("src/main.rs", "fn main() {}")
|
|
.build();
|
|
|
|
p.cargo("generate-lockfile -Zdirect-minimal-versions")
|
|
.masquerade_as_nightly_cargo(&["direct-minimal-versions"])
|
|
.with_status(101)
|
|
.with_stderr(
|
|
r#"[UPDATING] [..]
|
|
[ERROR] failed to select a version for `indirect`.
|
|
... required by package `direct v1.0.0`
|
|
... which satisfies dependency `direct = "^1.0"` of package `foo v0.0.1 ([CWD])`
|
|
versions that meet the requirements `^2.1` are: 2.2.0, 2.1.0
|
|
|
|
all possible versions conflict with previously selected packages.
|
|
|
|
previously selected package `indirect v2.0.0`
|
|
... which satisfies dependency `indirect = "^2.0"` of package `foo v0.0.1 ([CWD])`
|
|
|
|
failed to select a version for `indirect` which could resolve this conflict
|
|
"#,
|
|
)
|
|
.run();
|
|
}
|