mirror of
https://github.com/rust-lang/cargo
synced 2024-11-05 18:50:39 +00:00
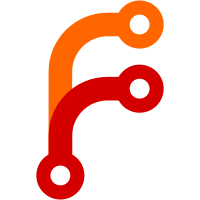
Keep deterministic builds for Cargo! The changes here are: * Sort files being added to the archive to ensure they're added in the same order on all platforms. * Flag the archive builder as "deterministic mode" which means it won't pick up fields like mtime. Closes #8599
39 lines
1.2 KiB
Rust
39 lines
1.2 KiB
Rust
use flate2::{Compression, GzBuilder};
|
|
use std::ffi::OsStr;
|
|
use std::fs;
|
|
use std::path::Path;
|
|
|
|
fn main() {
|
|
compress_man();
|
|
}
|
|
|
|
fn compress_man() {
|
|
let out_path = Path::new(&std::env::var("OUT_DIR").unwrap()).join("man.tgz");
|
|
let dst = fs::File::create(out_path).unwrap();
|
|
let encoder = GzBuilder::new()
|
|
.filename("man.tar")
|
|
.write(dst, Compression::best());
|
|
let mut ar = tar::Builder::new(encoder);
|
|
ar.mode(tar::HeaderMode::Deterministic);
|
|
|
|
let mut add_files = |dir, extension| {
|
|
let mut files = fs::read_dir(dir)
|
|
.unwrap()
|
|
.map(|e| e.unwrap().path())
|
|
.collect::<Vec<_>>();
|
|
files.sort();
|
|
for path in files {
|
|
if path.extension() != Some(extension) {
|
|
continue;
|
|
}
|
|
println!("cargo:rerun-if-changed={}", path.display());
|
|
ar.append_path_with_name(&path, path.file_name().unwrap())
|
|
.unwrap();
|
|
}
|
|
};
|
|
|
|
add_files(Path::new("src/etc/man"), OsStr::new("1"));
|
|
add_files(Path::new("src/doc/man/generated_txt"), OsStr::new("txt"));
|
|
let encoder = ar.into_inner().unwrap();
|
|
encoder.finish().unwrap();
|
|
}
|