mirror of
https://github.com/containers/podman
synced 2024-10-20 17:23:30 +00:00
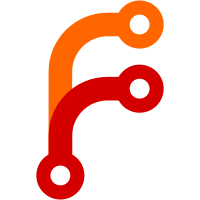
Migrate the diff, exec, export, and history bats tests to the ginkgo test suite. Signed-off-by: baude <bbaude@redhat.com> Closes: #287 Approved by: baude
63 lines
1.4 KiB
Go
63 lines
1.4 KiB
Go
package integration
|
|
|
|
import (
|
|
"os"
|
|
|
|
. "github.com/onsi/ginkgo"
|
|
. "github.com/onsi/gomega"
|
|
)
|
|
|
|
var _ = Describe("Podman exec", func() {
|
|
var (
|
|
tempdir string
|
|
err error
|
|
podmanTest PodmanTest
|
|
)
|
|
|
|
BeforeEach(func() {
|
|
tempdir, err = CreateTempDirInTempDir()
|
|
if err != nil {
|
|
os.Exit(1)
|
|
}
|
|
podmanTest = PodmanCreate(tempdir)
|
|
podmanTest.RestoreAllArtifacts()
|
|
})
|
|
|
|
AfterEach(func() {
|
|
podmanTest.Cleanup()
|
|
|
|
})
|
|
|
|
It("podman exec into bogus container", func() {
|
|
session := podmanTest.Podman([]string{"exec", "foobar", "ls"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(125))
|
|
})
|
|
|
|
It("podman exec without command", func() {
|
|
session := podmanTest.Podman([]string{"exec", "foobar"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(125))
|
|
})
|
|
|
|
It("podman exec simple command", func() {
|
|
setup := podmanTest.RunSleepContainer("test1")
|
|
setup.WaitWithDefaultTimeout()
|
|
Expect(setup.ExitCode()).To(Equal(0))
|
|
|
|
session := podmanTest.Podman([]string{"exec", "test1", "ls"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
})
|
|
|
|
It("podman exec simple command using latest", func() {
|
|
setup := podmanTest.RunSleepContainer("test1")
|
|
setup.WaitWithDefaultTimeout()
|
|
Expect(setup.ExitCode()).To(Equal(0))
|
|
|
|
session := podmanTest.Podman([]string{"exec", "-l", "ls"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
})
|
|
})
|