mirror of
https://github.com/containers/podman
synced 2024-10-19 16:54:07 +00:00
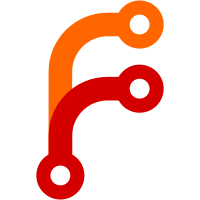
Automated for .go files via gomove [1]: `gomove github.com/containers/podman/v3 github.com/containers/podman/v4` Remaining files via vgrep [2]: `vgrep github.com/containers/podman/v3` [1] https://github.com/KSubedi/gomove [2] https://github.com/vrothberg/vgrep Signed-off-by: Valentin Rothberg <rothberg@redhat.com>
62 lines
1.8 KiB
Go
62 lines
1.8 KiB
Go
package libpod
|
|
|
|
import "github.com/containers/podman/v4/libpod/define"
|
|
|
|
// GetPodStatus determines the status of the pod based on the
|
|
// statuses of the containers in the pod.
|
|
// Returns a string representation of the pod status
|
|
func (p *Pod) GetPodStatus() (string, error) {
|
|
ctrStatuses, err := p.Status()
|
|
if err != nil {
|
|
return define.PodStateErrored, err
|
|
}
|
|
return createPodStatusResults(ctrStatuses)
|
|
}
|
|
|
|
func createPodStatusResults(ctrStatuses map[string]define.ContainerStatus) (string, error) {
|
|
ctrNum := len(ctrStatuses)
|
|
if ctrNum == 0 {
|
|
return define.PodStateCreated, nil
|
|
}
|
|
statuses := map[string]int{
|
|
define.PodStateStopped: 0,
|
|
define.PodStateRunning: 0,
|
|
define.PodStatePaused: 0,
|
|
define.PodStateCreated: 0,
|
|
define.PodStateErrored: 0,
|
|
}
|
|
for _, ctrStatus := range ctrStatuses {
|
|
switch ctrStatus {
|
|
case define.ContainerStateExited:
|
|
fallthrough
|
|
case define.ContainerStateStopped:
|
|
statuses[define.PodStateStopped]++
|
|
case define.ContainerStateRunning:
|
|
statuses[define.PodStateRunning]++
|
|
case define.ContainerStatePaused:
|
|
statuses[define.PodStatePaused]++
|
|
case define.ContainerStateCreated, define.ContainerStateConfigured:
|
|
statuses[define.PodStateCreated]++
|
|
default:
|
|
statuses[define.PodStateErrored]++
|
|
}
|
|
}
|
|
|
|
switch {
|
|
case statuses[define.PodStateRunning] == ctrNum:
|
|
return define.PodStateRunning, nil
|
|
case statuses[define.PodStateRunning] > 0:
|
|
return define.PodStateDegraded, nil
|
|
case statuses[define.PodStatePaused] == ctrNum:
|
|
return define.PodStatePaused, nil
|
|
case statuses[define.PodStateStopped] == ctrNum:
|
|
return define.PodStateExited, nil
|
|
case statuses[define.PodStateStopped] > 0:
|
|
return define.PodStateStopped, nil
|
|
case statuses[define.PodStateErrored] > 0:
|
|
return define.PodStateErrored, nil
|
|
default:
|
|
return define.PodStateCreated, nil
|
|
}
|
|
}
|