mirror of
https://github.com/containers/podman
synced 2024-10-20 17:23:30 +00:00
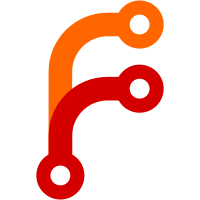
Reimplement CI-automation to remove accumulated technical-debt and optimize workflow. The task-dependency graph designed goal was to shorten it's depth and increase width (i.e. more parallelism). A reduction in redundant building (and 3rd party module download) was also realized by caching `$GOPATH` and `$GOCACHE` early on. This cache is then reused in favor of a fresh clone of the repository (when possible). Note: The system tests typically execute MUCH faster than the integration tests. However, contrary to a fail-fast/fail-early principal, they are executed last. This was implemented due to debug-ability related concerns/preferences of the primary (golang-centric) project developers. Signed-off-by: Chris Evich <cevich@redhat.com>
63 lines
2 KiB
Python
Executable file
63 lines
2 KiB
Python
Executable file
#!/usr/bin/env python3
|
|
|
|
"""
|
|
Verify contents of .cirrus.yml meet specific expectations
|
|
"""
|
|
|
|
import sys
|
|
import os
|
|
import unittest
|
|
import yaml
|
|
|
|
# Assumes directory structure of this file relative to repo.
|
|
SCRIPT_DIRPATH = os.path.dirname(os.path.realpath(__file__))
|
|
REPO_ROOT = os.path.realpath(os.path.join(SCRIPT_DIRPATH, '../', '../'))
|
|
|
|
|
|
class TestCaseBase(unittest.TestCase):
|
|
|
|
CIRRUS_YAML = None
|
|
|
|
def setUp(self):
|
|
with open(os.path.join(REPO_ROOT, '.cirrus.yml')) as cirrus_yaml:
|
|
self.CIRRUS_YAML = yaml.safe_load(cirrus_yaml.read())
|
|
|
|
|
|
class TestDependsOn(TestCaseBase):
|
|
|
|
ALL_TASK_NAMES = None
|
|
SUCCESS_DEPS_EXCLUDE = set(['success', 'release', 'release_test'])
|
|
|
|
def setUp(self):
|
|
super().setUp()
|
|
self.ALL_TASK_NAMES = set([key.replace('_task', '')
|
|
for key, _ in self.CIRRUS_YAML.items()
|
|
if key.endswith('_task')])
|
|
|
|
def test_dicts(self):
|
|
"""Expected dictionaries are present and non-empty"""
|
|
self.assertIn('success_task', self.CIRRUS_YAML)
|
|
self.assertIn('success_task'.replace('_task', ''), self.ALL_TASK_NAMES)
|
|
self.assertIn('depends_on', self.CIRRUS_YAML['success_task'])
|
|
self.assertGreater(len(self.CIRRUS_YAML['success_task']['depends_on']), 0)
|
|
|
|
def test_task(self):
|
|
"""There is no task named 'task'"""
|
|
self.assertNotIn('task', self.ALL_TASK_NAMES)
|
|
|
|
def test_depends(self):
|
|
"""Success task depends on all other tasks"""
|
|
success_deps = set(self.CIRRUS_YAML['success_task']['depends_on'])
|
|
for task_name in self.ALL_TASK_NAMES - self.SUCCESS_DEPS_EXCLUDE:
|
|
with self.subTest(task_name=task_name):
|
|
msg=('Please add "{0}" to the "depends_on" list in "success_task"'
|
|
"".format(task_name))
|
|
self.assertIn(task_name, success_deps, msg=msg)
|
|
|
|
def not_task(self):
|
|
"""Ensure no task is named 'task'"""
|
|
self.assertNotIn('task', self.ALL_TASK_NAMES)
|
|
|
|
if __name__ == "__main__":
|
|
unittest.main()
|