mirror of
https://github.com/containers/podman
synced 2024-10-20 17:23:30 +00:00
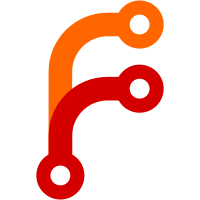
Some CI systems set $OCI_RUNTIME as a way to override the default crun. Integration (e2e) tests honor this, but system tests were not aware of the convention; this means we haven't been testing system tests with runc, which means RHEL gating tests are now failing. The proper solution would be to edit containers.conf on CI systems. Sorry, that would involve too much CI-VM work. Instead, this PR detects $OCI_RUNTIME and creates a dummy containers.conf file using that runtime. Add: various skips for tests that don't work with runc. Refactor: add a helper function so we don't need to do the complicated 'podman info blah blah .OCIRuntime.blah' thing in many places. BUG: we leave a tmp file behind on exit. Signed-off-by: Ed Santiago <santiago@redhat.com>
86 lines
2.5 KiB
Bash
86 lines
2.5 KiB
Bash
#!/usr/bin/env bats
|
|
|
|
load helpers
|
|
|
|
@test "podman info - basic test" {
|
|
run_podman info
|
|
|
|
expected_keys="
|
|
buildahVersion: *[0-9.]\\\+
|
|
conmon:\\\s\\\+package:
|
|
distribution:
|
|
ociRuntime:\\\s\\\+name:
|
|
os:
|
|
rootless:
|
|
registries:
|
|
store:
|
|
graphDriverName:
|
|
graphRoot:
|
|
graphStatus:
|
|
imageStore:\\\s\\\+number: 1
|
|
runRoot:
|
|
cgroupManager: \\\(systemd\\\|cgroupfs\\\)
|
|
cgroupVersion: v[12]
|
|
"
|
|
while read expect; do
|
|
is "$output" ".*$expect" "output includes '$expect'"
|
|
done < <(parse_table "$expected_keys")
|
|
}
|
|
|
|
@test "podman info - json" {
|
|
run_podman info --format=json
|
|
|
|
expr_nvr="[a-z0-9-]\\\+-[a-z0-9.]\\\+-[a-z0-9]\\\+\."
|
|
expr_path="/[a-z0-9\\\/.-]\\\+\\\$"
|
|
|
|
tests="
|
|
host.buildahVersion | [0-9.]
|
|
host.conmon.path | $expr_path
|
|
host.cgroupManager | \\\(systemd\\\|cgroupfs\\\)
|
|
host.cgroupVersion | v[12]
|
|
host.ociRuntime.path | $expr_path
|
|
store.configFile | $expr_path
|
|
store.graphDriverName | [a-z0-9]\\\+\\\$
|
|
store.graphRoot | $expr_path
|
|
store.imageStore.number | 1
|
|
"
|
|
|
|
parse_table "$tests" | while read field expect; do
|
|
actual=$(echo "$output" | jq -r ".$field")
|
|
dprint "# actual=<$actual> expect=<$expect>"
|
|
is "$actual" "$expect" "jq .$field"
|
|
done
|
|
|
|
}
|
|
|
|
# 2021-04-06 discussed in watercooler: RHEL must never use crun, even if
|
|
# using cgroups v2.
|
|
@test "podman info - RHEL8 must use runc" {
|
|
local osrelease=/etc/os-release
|
|
test -e $osrelease || skip "Not a RHEL system (no $osrelease)"
|
|
|
|
local osname=$(source $osrelease; echo $NAME)
|
|
if [[ $osname =~ Red.Hat || $osname =~ CentOS ]]; then
|
|
# Version can include minor; strip off first dot an all beyond it
|
|
local osver=$(source $osrelease; echo $VERSION_ID)
|
|
test ${osver%%.*} -le 8 || skip "$osname $osver > RHEL8"
|
|
|
|
# RHEL or CentOS 8.
|
|
# FIXME: what does 'CentOS 8' even mean? What is $VERSION_ID in CentOS?
|
|
is "$(podman_runtime)" "runc" "$osname only supports OCI Runtime = runc"
|
|
else
|
|
skip "only applicable on RHEL, this is $osname"
|
|
fi
|
|
}
|
|
|
|
@test "podman info --storage-opt='' " {
|
|
skip_if_remote "--storage-opt flag is not supported for remote"
|
|
skip_if_rootless "storage opts are required for rootless running"
|
|
run_podman --storage-opt='' info
|
|
# Note this will not work in rootless mode, unless you specify
|
|
# storage-driver=vfs, until we have kernels that support rootless overlay
|
|
# mounts.
|
|
is "$output" ".*graphOptions: {}" "output includes graphOptions: {}"
|
|
}
|
|
# vim: filetype=sh
|