mirror of
https://github.com/containers/podman
synced 2024-10-20 17:23:30 +00:00
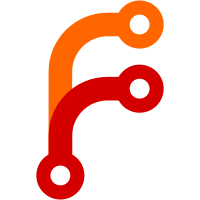
Updating the vendor or runc to pull in some fixes that we need. In order to get this vendor to work, we needed to update the vendor of docker/docker, which causes all sorts of issues, just to fix the docker/pkg/sysinfo. Rather then doing this, I pulled in pkg/sysinfo into libpod and fixed the code locally. I then switched the use of docker/pkg/sysinfo to libpod/pkg/sysinfo. I also switched out the docker/pkg/mount to containers/storage/pkg/mount Signed-off-by: Daniel J Walsh <dwalsh@redhat.com>
145 lines
3.7 KiB
Go
145 lines
3.7 KiB
Go
package sysinfo
|
|
|
|
import "github.com/docker/docker/pkg/parsers"
|
|
|
|
// SysInfo stores information about which features a kernel supports.
|
|
// TODO Windows: Factor out platform specific capabilities.
|
|
type SysInfo struct {
|
|
// Whether the kernel supports AppArmor or not
|
|
AppArmor bool
|
|
// Whether the kernel supports Seccomp or not
|
|
Seccomp bool
|
|
|
|
cgroupMemInfo
|
|
cgroupCPUInfo
|
|
cgroupBlkioInfo
|
|
cgroupCpusetInfo
|
|
cgroupPids
|
|
|
|
// Whether IPv4 forwarding is supported or not, if this was disabled, networking will not work
|
|
IPv4ForwardingDisabled bool
|
|
|
|
// Whether bridge-nf-call-iptables is supported or not
|
|
BridgeNFCallIPTablesDisabled bool
|
|
|
|
// Whether bridge-nf-call-ip6tables is supported or not
|
|
BridgeNFCallIP6TablesDisabled bool
|
|
|
|
// Whether the cgroup has the mountpoint of "devices" or not
|
|
CgroupDevicesEnabled bool
|
|
}
|
|
|
|
type cgroupMemInfo struct {
|
|
// Whether memory limit is supported or not
|
|
MemoryLimit bool
|
|
|
|
// Whether swap limit is supported or not
|
|
SwapLimit bool
|
|
|
|
// Whether soft limit is supported or not
|
|
MemoryReservation bool
|
|
|
|
// Whether OOM killer disable is supported or not
|
|
OomKillDisable bool
|
|
|
|
// Whether memory swappiness is supported or not
|
|
MemorySwappiness bool
|
|
|
|
// Whether kernel memory limit is supported or not
|
|
KernelMemory bool
|
|
}
|
|
|
|
type cgroupCPUInfo struct {
|
|
// Whether CPU shares is supported or not
|
|
CPUShares bool
|
|
|
|
// Whether CPU CFS(Completely Fair Scheduler) period is supported or not
|
|
CPUCfsPeriod bool
|
|
|
|
// Whether CPU CFS(Completely Fair Scheduler) quota is supported or not
|
|
CPUCfsQuota bool
|
|
|
|
// Whether CPU real-time period is supported or not
|
|
CPURealtimePeriod bool
|
|
|
|
// Whether CPU real-time runtime is supported or not
|
|
CPURealtimeRuntime bool
|
|
}
|
|
|
|
type cgroupBlkioInfo struct {
|
|
// Whether Block IO weight is supported or not
|
|
BlkioWeight bool
|
|
|
|
// Whether Block IO weight_device is supported or not
|
|
BlkioWeightDevice bool
|
|
|
|
// Whether Block IO read limit in bytes per second is supported or not
|
|
BlkioReadBpsDevice bool
|
|
|
|
// Whether Block IO write limit in bytes per second is supported or not
|
|
BlkioWriteBpsDevice bool
|
|
|
|
// Whether Block IO read limit in IO per second is supported or not
|
|
BlkioReadIOpsDevice bool
|
|
|
|
// Whether Block IO write limit in IO per second is supported or not
|
|
BlkioWriteIOpsDevice bool
|
|
}
|
|
|
|
type cgroupCpusetInfo struct {
|
|
// Whether Cpuset is supported or not
|
|
Cpuset bool
|
|
|
|
// Available Cpuset's cpus
|
|
Cpus string
|
|
|
|
// Available Cpuset's memory nodes
|
|
Mems string
|
|
}
|
|
|
|
type cgroupPids struct {
|
|
// Whether Pids Limit is supported or not
|
|
PidsLimit bool
|
|
}
|
|
|
|
// IsCpusetCpusAvailable returns `true` if the provided string set is contained
|
|
// in cgroup's cpuset.cpus set, `false` otherwise.
|
|
// If error is not nil a parsing error occurred.
|
|
func (c cgroupCpusetInfo) IsCpusetCpusAvailable(provided string) (bool, error) {
|
|
return isCpusetListAvailable(provided, c.Cpus)
|
|
}
|
|
|
|
// IsCpusetMemsAvailable returns `true` if the provided string set is contained
|
|
// in cgroup's cpuset.mems set, `false` otherwise.
|
|
// If error is not nil a parsing error occurred.
|
|
func (c cgroupCpusetInfo) IsCpusetMemsAvailable(provided string) (bool, error) {
|
|
return isCpusetListAvailable(provided, c.Mems)
|
|
}
|
|
|
|
func isCpusetListAvailable(provided, available string) (bool, error) {
|
|
parsedProvided, err := parsers.ParseUintList(provided)
|
|
if err != nil {
|
|
return false, err
|
|
}
|
|
parsedAvailable, err := parsers.ParseUintList(available)
|
|
if err != nil {
|
|
return false, err
|
|
}
|
|
for k := range parsedProvided {
|
|
if !parsedAvailable[k] {
|
|
return false, nil
|
|
}
|
|
}
|
|
return true, nil
|
|
}
|
|
|
|
// Returns bit count of 1, used by NumCPU
|
|
func popcnt(x uint64) (n byte) {
|
|
x -= (x >> 1) & 0x5555555555555555
|
|
x = (x>>2)&0x3333333333333333 + x&0x3333333333333333
|
|
x += x >> 4
|
|
x &= 0x0f0f0f0f0f0f0f0f
|
|
x *= 0x0101010101010101
|
|
return byte(x >> 56)
|
|
}
|