mirror of
https://github.com/containers/podman
synced 2024-10-19 16:54:07 +00:00
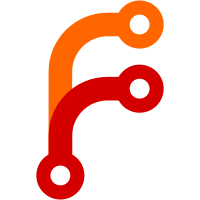
the compilation demands of having libpod in main is a burden for the remote client compilations. to combat this, we should move the use of libpod structs, vars, constants, and functions into the adapter code where it will only be compiled by the local client. this should result in cleaner code organization and smaller binaries. it should also help if we ever need to compile the remote client on non-Linux operating systems natively (not cross-compiled). Signed-off-by: baude <bbaude@redhat.com>
87 lines
2.2 KiB
Go
87 lines
2.2 KiB
Go
package libpod
|
|
|
|
import (
|
|
"fmt"
|
|
"path/filepath"
|
|
"time"
|
|
|
|
"github.com/containers/libpod/libpod/define"
|
|
"github.com/containers/storage/pkg/stringid"
|
|
"github.com/pkg/errors"
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
// Creates a new, empty pod
|
|
func newPod(runtime *Runtime) (*Pod, error) {
|
|
pod := new(Pod)
|
|
pod.config = new(PodConfig)
|
|
pod.config.ID = stringid.GenerateNonCryptoID()
|
|
pod.config.Labels = make(map[string]string)
|
|
pod.config.CreatedTime = time.Now()
|
|
pod.config.InfraContainer = new(InfraContainerConfig)
|
|
pod.state = new(podState)
|
|
pod.runtime = runtime
|
|
|
|
return pod, nil
|
|
}
|
|
|
|
// Update pod state from database
|
|
func (p *Pod) updatePod() error {
|
|
if err := p.runtime.state.UpdatePod(p); err != nil {
|
|
return err
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// Save pod state to database
|
|
func (p *Pod) save() error {
|
|
if err := p.runtime.state.SavePod(p); err != nil {
|
|
return errors.Wrapf(err, "error saving pod %s state", p.ID())
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// Refresh a pod's state after restart
|
|
// This cannot lock any other pod, but may lock individual containers, as those
|
|
// will have refreshed by the time pod refresh runs.
|
|
func (p *Pod) refresh() error {
|
|
// Need to to an update from the DB to pull potentially-missing state
|
|
if err := p.runtime.state.UpdatePod(p); err != nil {
|
|
return err
|
|
}
|
|
|
|
if !p.valid {
|
|
return define.ErrPodRemoved
|
|
}
|
|
|
|
// Retrieve the pod's lock
|
|
lock, err := p.runtime.lockManager.AllocateAndRetrieveLock(p.config.LockID)
|
|
if err != nil {
|
|
return errors.Wrapf(err, "error retrieving lock %d for pod %s", p.config.LockID, p.ID())
|
|
}
|
|
p.lock = lock
|
|
|
|
// We need to recreate the pod's cgroup
|
|
if p.config.UsePodCgroup {
|
|
switch p.runtime.config.CgroupManager {
|
|
case SystemdCgroupsManager:
|
|
cgroupPath, err := systemdSliceFromPath(p.config.CgroupParent, fmt.Sprintf("libpod_pod_%s", p.ID()))
|
|
if err != nil {
|
|
logrus.Errorf("Error creating CGroup for pod %s: %v", p.ID(), err)
|
|
}
|
|
p.state.CgroupPath = cgroupPath
|
|
case CgroupfsCgroupsManager:
|
|
p.state.CgroupPath = filepath.Join(p.config.CgroupParent, p.ID())
|
|
|
|
logrus.Debugf("setting pod cgroup to %s", p.state.CgroupPath)
|
|
default:
|
|
return errors.Wrapf(define.ErrInvalidArg, "unknown cgroups manager %s specified", p.runtime.config.CgroupManager)
|
|
}
|
|
}
|
|
|
|
// Save changes
|
|
return p.save()
|
|
}
|