mirror of
https://github.com/containers/podman
synced 2024-10-20 17:23:30 +00:00
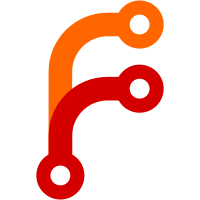
Also start using podmin in /usr/libexec/podman rather then crio. Signed-off-by: Daniel J Walsh <dwalsh@redhat.com> Closes: #979 Approved by: baude
69 lines
1.9 KiB
Go
69 lines
1.9 KiB
Go
package integration
|
|
|
|
import (
|
|
"os"
|
|
|
|
. "github.com/onsi/ginkgo"
|
|
. "github.com/onsi/gomega"
|
|
)
|
|
|
|
var _ = Describe("Podman run ns", func() {
|
|
var (
|
|
tempdir string
|
|
err error
|
|
podmanTest PodmanTest
|
|
)
|
|
|
|
BeforeEach(func() {
|
|
tempdir, err = CreateTempDirInTempDir()
|
|
if err != nil {
|
|
os.Exit(1)
|
|
}
|
|
podmanTest = PodmanCreate(tempdir)
|
|
podmanTest.RestoreAllArtifacts()
|
|
podmanTest.RestoreArtifact(fedoraMinimal)
|
|
})
|
|
|
|
AfterEach(func() {
|
|
podmanTest.Cleanup()
|
|
|
|
})
|
|
|
|
It("podman run pidns test", func() {
|
|
session := podmanTest.Podman([]string{"run", fedoraMinimal, "bash", "-c", "echo $$"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
Expect(session.OutputToString()).To(Equal("1"))
|
|
|
|
session = podmanTest.Podman([]string{"run", "--pid=host", fedoraMinimal, "bash", "-c", "echo $$"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
Expect(session.OutputToString()).To(Not(Equal("1")))
|
|
|
|
session = podmanTest.Podman([]string{"run", "--pid=badpid", fedoraMinimal, "bash", "-c", "echo $$"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Not(Equal(0)))
|
|
})
|
|
|
|
It("podman run ipcns test", func() {
|
|
testFile := "/dev/shm/podmantest"
|
|
setup := podmanTest.SystemExec("touch", []string{testFile})
|
|
setup.WaitWithDefaultTimeout()
|
|
Expect(setup.ExitCode()).To(Equal(0))
|
|
|
|
session := podmanTest.Podman([]string{"run", "--ipc=host", fedoraMinimal, "ls", testFile})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
Expect(session.OutputToString()).To(ContainSubstring(testFile))
|
|
|
|
err := os.Remove(testFile)
|
|
Expect(err).To(BeNil())
|
|
})
|
|
|
|
It("podman run bad ipc pid test", func() {
|
|
session := podmanTest.Podman([]string{"run", "--ipc=badpid", fedoraMinimal, "bash", "-c", "echo $$"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).ToNot(Equal(0))
|
|
})
|
|
})
|