mirror of
https://github.com/containers/podman
synced 2024-10-20 09:13:46 +00:00
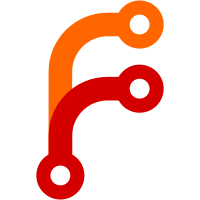
Previously, we only did this for volumes created at the same time as the container. However, this is not correct behavior - Docker does so for all named volumes, even those made with 'podman volume create' and mounted into a container later. Fixes #3945 Signed-off-by: Matthew Heon <matthew.heon@pm.me>
49 lines
1.2 KiB
Go
49 lines
1.2 KiB
Go
package libpod
|
|
|
|
import (
|
|
"os"
|
|
"path/filepath"
|
|
|
|
"github.com/containers/libpod/libpod/define"
|
|
)
|
|
|
|
// Creates a new volume
|
|
func newVolume(runtime *Runtime) (*Volume, error) {
|
|
volume := new(Volume)
|
|
volume.config = new(VolumeConfig)
|
|
volume.state = new(VolumeState)
|
|
volume.runtime = runtime
|
|
volume.config.Labels = make(map[string]string)
|
|
volume.config.Options = make(map[string]string)
|
|
volume.state.NeedsCopyUp = true
|
|
|
|
return volume, nil
|
|
}
|
|
|
|
// teardownStorage deletes the volume from volumePath
|
|
func (v *Volume) teardownStorage() error {
|
|
return os.RemoveAll(filepath.Join(v.runtime.config.VolumePath, v.Name()))
|
|
}
|
|
|
|
// Volumes with options set, or a filesystem type, or a device to mount need to
|
|
// be mounted and unmounted.
|
|
func (v *Volume) needsMount() bool {
|
|
return len(v.config.Options) > 0 && v.config.Driver == define.VolumeDriverLocal
|
|
}
|
|
|
|
// update() updates the volume state from the DB.
|
|
func (v *Volume) update() error {
|
|
if err := v.runtime.state.UpdateVolume(v); err != nil {
|
|
return err
|
|
}
|
|
if !v.valid {
|
|
return define.ErrVolumeRemoved
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// save() saves the volume state to the DB
|
|
func (v *Volume) save() error {
|
|
return v.runtime.state.SaveVolume(v)
|
|
}
|