mirror of
https://github.com/containers/podman
synced 2024-10-19 16:54:07 +00:00
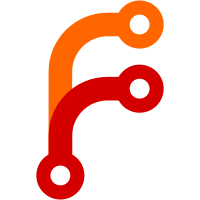
The BATS 'run' directive is really quite obnoxious; for the most part we really don't want to use it. Remove some uses that snuck in last week, and remove one test (exists) that can more naturally be piggybacked into an rm test. While we're at it: in setup(), look for and delete stray external (buildah) containers. This will be important if any of the external-container tests fails; this way we don't leave behind a state that causes subsequent tests to fail. Signed-off-by: Ed Santiago <santiago@redhat.com>
71 lines
2.2 KiB
Bash
71 lines
2.2 KiB
Bash
#!/usr/bin/env bats -*- bats -*-
|
|
#
|
|
# tests for podman rm
|
|
#
|
|
|
|
load helpers
|
|
|
|
@test "podman rm" {
|
|
rand=$(random_string 30)
|
|
run_podman run --name $rand $IMAGE /bin/true
|
|
|
|
# Don't care about output, just check exit status (it should exist)
|
|
run_podman 0 inspect $rand
|
|
|
|
# container should be in output of 'ps -a'
|
|
run_podman ps -a
|
|
is "$output" ".* $IMAGE .*/true .* $rand" "Container present in 'ps -a'"
|
|
|
|
# Remove container; now 'inspect' should fail
|
|
run_podman rm $rand
|
|
run_podman 125 inspect $rand
|
|
}
|
|
|
|
@test "podman rm - running container, w/o and w/ force" {
|
|
run_podman run -d $IMAGE sleep 5
|
|
cid="$output"
|
|
|
|
# rm should fail
|
|
run_podman 2 rm $cid
|
|
is "$output" "Error: cannot remove container $cid as it is running - running or paused containers cannot be removed without force: container state improper" "error message"
|
|
|
|
# rm -f should succeed
|
|
run_podman rm -f $cid
|
|
}
|
|
|
|
@test "podman rm container from storage" {
|
|
if is_remote; then
|
|
skip "only applicable for local podman"
|
|
fi
|
|
rand=$(random_string 30)
|
|
run_podman create --name $rand $IMAGE /bin/true
|
|
|
|
# Create a container that podman does not know about
|
|
external_cid=$(buildah from $IMAGE)
|
|
|
|
# Plain 'exists' should fail, but should succeed with --external
|
|
run_podman 1 container exists $external_cid
|
|
run_podman container exists --external $external_cid
|
|
|
|
# rm should succeed
|
|
run_podman rm $rand $external_cid
|
|
}
|
|
|
|
# I'm sorry! This test takes 13 seconds. There's not much I can do about it,
|
|
# please know that I think it's justified: podman 1.5.0 had a strange bug
|
|
# in with exit status was not preserved on some code paths with 'rm -f'
|
|
# or 'podman run --rm' (see also 030-run.bats). The test below is a bit
|
|
# kludgy: what we care about is the exit status of the killed container,
|
|
# not 'podman rm', but BATS has no provision (that I know of) for forking,
|
|
# so what we do is start the 'rm' beforehand and monitor the exit status
|
|
# of the 'sleep' container.
|
|
#
|
|
# See https://github.com/containers/podman/issues/3795
|
|
@test "podman rm -f" {
|
|
rand=$(random_string 30)
|
|
( sleep 3; run_podman rm -f $rand ) &
|
|
run_podman 137 run --name $rand $IMAGE sleep 30
|
|
}
|
|
|
|
# vim: filetype=sh
|