mirror of
https://github.com/containers/podman
synced 2024-10-19 08:44:11 +00:00
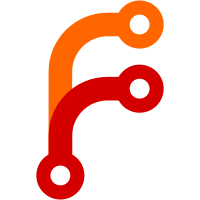
We missed bumping the go module, so let's do it now :) * Automated go code with github.com/sirkon/go-imports-rename * Manually via `vgrep podman/v2` the rest Signed-off-by: Valentin Rothberg <rothberg@redhat.com>
156 lines
4.5 KiB
Go
156 lines
4.5 KiB
Go
package integration
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
|
|
. "github.com/containers/podman/v3/test/utils"
|
|
. "github.com/onsi/ginkgo"
|
|
. "github.com/onsi/gomega"
|
|
)
|
|
|
|
var _ = Describe("Podman pod kill", func() {
|
|
var (
|
|
tempdir string
|
|
err error
|
|
podmanTest *PodmanTestIntegration
|
|
)
|
|
|
|
BeforeEach(func() {
|
|
tempdir, err = CreateTempDirInTempDir()
|
|
if err != nil {
|
|
os.Exit(1)
|
|
}
|
|
podmanTest = PodmanTestCreate(tempdir)
|
|
podmanTest.Setup()
|
|
podmanTest.SeedImages()
|
|
})
|
|
|
|
AfterEach(func() {
|
|
podmanTest.Cleanup()
|
|
f := CurrentGinkgoTestDescription()
|
|
processTestResult(f)
|
|
|
|
})
|
|
|
|
It("podman pod kill bogus", func() {
|
|
session := podmanTest.Podman([]string{"pod", "kill", "foobar"})
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session).To(ExitWithError())
|
|
})
|
|
|
|
It("podman pod kill a pod by id", func() {
|
|
_, ec, podid := podmanTest.CreatePod(nil)
|
|
Expect(ec).To(Equal(0))
|
|
|
|
session := podmanTest.RunTopContainerInPod("", podid)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
|
|
session = podmanTest.RunTopContainerInPod("", podid)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
|
|
result := podmanTest.Podman([]string{"pod", "kill", podid})
|
|
result.WaitWithDefaultTimeout()
|
|
Expect(result.ExitCode()).To(Equal(0))
|
|
Expect(podmanTest.NumberOfContainersRunning()).To(Equal(0))
|
|
})
|
|
|
|
It("podman pod kill a pod by id with TERM", func() {
|
|
_, ec, podid := podmanTest.CreatePod(nil)
|
|
Expect(ec).To(Equal(0))
|
|
|
|
session := podmanTest.RunTopContainerInPod("", podid)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
|
|
result := podmanTest.Podman([]string{"pod", "kill", "-s", "9", podid})
|
|
result.WaitWithDefaultTimeout()
|
|
Expect(result.ExitCode()).To(Equal(0))
|
|
Expect(podmanTest.NumberOfContainersRunning()).To(Equal(0))
|
|
})
|
|
|
|
It("podman pod kill a pod by name", func() {
|
|
_, ec, podid := podmanTest.CreatePod(map[string][]string{"--name": {"test1"}})
|
|
Expect(ec).To(Equal(0))
|
|
|
|
session := podmanTest.RunTopContainerInPod("", podid)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
|
|
result := podmanTest.Podman([]string{"pod", "kill", "test1"})
|
|
result.WaitWithDefaultTimeout()
|
|
Expect(result.ExitCode()).To(Equal(0))
|
|
Expect(podmanTest.NumberOfContainersRunning()).To(Equal(0))
|
|
})
|
|
|
|
It("podman pod kill a pod by id with a bogus signal", func() {
|
|
_, ec, podid := podmanTest.CreatePod(map[string][]string{"--name": {"test1"}})
|
|
Expect(ec).To(Equal(0))
|
|
|
|
session := podmanTest.RunTopContainerInPod("", podid)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
|
|
result := podmanTest.Podman([]string{"pod", "kill", "-s", "bogus", "test1"})
|
|
result.WaitWithDefaultTimeout()
|
|
Expect(result.ExitCode()).To(Equal(125))
|
|
Expect(podmanTest.NumberOfContainersRunning()).To(Equal(1))
|
|
})
|
|
|
|
It("podman pod kill latest pod", func() {
|
|
_, ec, podid := podmanTest.CreatePod(nil)
|
|
Expect(ec).To(Equal(0))
|
|
|
|
session := podmanTest.RunTopContainerInPod("", podid)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
|
|
_, ec, podid2 := podmanTest.CreatePod(nil)
|
|
Expect(ec).To(Equal(0))
|
|
|
|
session = podmanTest.RunTopContainerInPod("", podid2)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
|
|
session = podmanTest.RunTopContainerInPod("", podid2)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
if !IsRemote() {
|
|
podid2 = "-l"
|
|
}
|
|
result := podmanTest.Podman([]string{"pod", "kill", podid2})
|
|
result.WaitWithDefaultTimeout()
|
|
Expect(result.ExitCode()).To(Equal(0))
|
|
Expect(podmanTest.NumberOfContainersRunning()).To(Equal(1))
|
|
})
|
|
|
|
It("podman pod kill all", func() {
|
|
SkipIfRootlessCgroupsV1("Not supported for rootless + CGroupsV1")
|
|
_, ec, podid := podmanTest.CreatePod(nil)
|
|
Expect(ec).To(Equal(0))
|
|
|
|
session := podmanTest.RunTopContainerInPod("", podid)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
|
|
session = podmanTest.RunTopContainerInPod("", podid)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
|
|
_, ec, podid2 := podmanTest.CreatePod(nil)
|
|
Expect(ec).To(Equal(0))
|
|
|
|
session = podmanTest.RunTopContainerInPod("", podid2)
|
|
session.WaitWithDefaultTimeout()
|
|
Expect(session.ExitCode()).To(Equal(0))
|
|
|
|
result := podmanTest.Podman([]string{"pod", "kill", "-a"})
|
|
result.WaitWithDefaultTimeout()
|
|
fmt.Println(result.OutputToString(), result.ErrorToString())
|
|
Expect(result.ExitCode()).To(Equal(0))
|
|
Expect(podmanTest.NumberOfContainersRunning()).To(Equal(0))
|
|
})
|
|
})
|